The Visitor pattern
We have a FamilyMember hierarchy, as shown in the following figure, which is pretty stable. We have another hierarchy, Friends
, which is pretty open ended. So now, a FamilyMember needs to accept a Friend.
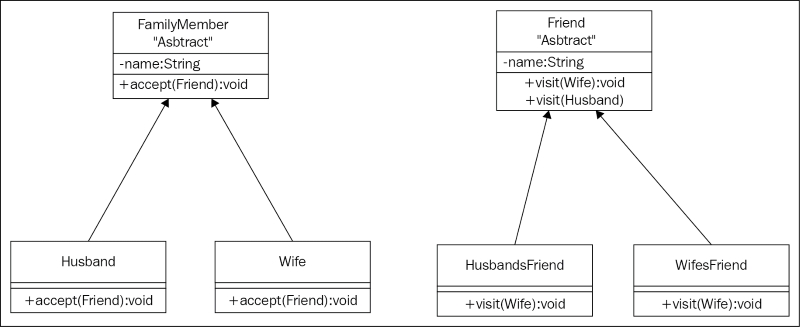
Figure 7.2: A UML diagram—Visitors
When we have a hierarchy and we need to add a new method to it without modifying it, we use the visitor pattern. Here is the code snippet for this example:
public abstract class FamilyMember { . private String name; public FamilyMember(String name) { this.name = name; } public String getName() { return name; } public abstract void accept(Friend friend); // 1 } public class Husband extends FamilyMember { public Husband(String name) { super(name); } @Override public void accept(Friend friend) { // 2 friend.greet(this); // 3 } }
The salient points of the preceding example are as follows:
Here, we have an
accept
method, accepting all friends. TheFamilyMember
hierarchy opens up its doors forFriends...