Hibernate's lazy loading
Hibernate exposes persistence-related database access via an object API. A Java class is mapped to a database table. A parent having many children is mapped as shown in the following code:
@Entity public class Parent { … @OneToMany(mappedBy = "parent") private Set<Child> children; … } @Entity public class Child { … @ManyToOne @JoinColumn(name = "parent_id") private Parent parent; … }
This maps the following table structure:
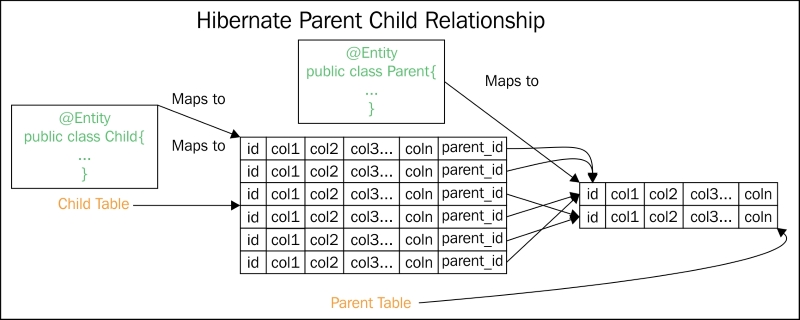
Figure 4.3: Proxying in Hibernate
The children are, by default, lazy loaded. This holds true for any mapped members that are collections. You may not need them all the time you load the parent. If you do, you can load them as needed. The set is actually a PersistenceSet, a proxy. The catch is that this is not completely transparent though. You need to have a hibernate session active to load the children. Otherwise, you get a LazyInitializationException
.
Why is this done? The children may have children of their own. The object graph, parent...