Implementing your own caching algorithm
In this section, let us start by implementing a simple cache algorithm and see its draw backs, and then show how spring caching can be used to solve the problems.
Let's draw a simple flow chart to look at the caching scenario:
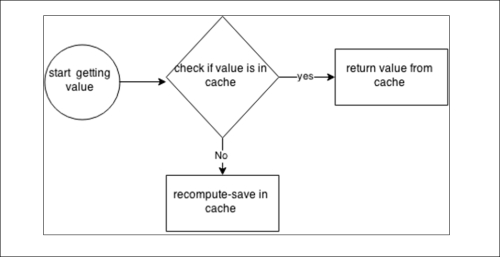
Let's see how we can implement caching in a simple way. Think of generating a Fibonacci number. A Fibonacci number is generated by adding its previous two Fibonacci numbers. So we can compute a simple class in java and see how we can use caching here.
Let's create a map to cache the objects:
import java.util.HashMap; import java.util.Map; public class FibonacciCache { private Map<Long, Long> cachemap = new HashMap<>(); public FibonacciCache() { // The base case for the Fibonacci Sequence cachemap.put(0L, 1L); cachemap.put(1L, 1L); } public Long getNumber(long index) { // Check if value is in cache if (cachemap.containsKey(index)) { return cachemap.get(index); } ...