Deleting rows from a list
So far, we've learned how to add new rows to a list. Now, let's find out how to use a swipe motion to delete items one at a time.
Getting ready
Create a new SwiftUI app called ListRowDelete
.
How to do it…
We will create a list of items and use the list view's onDelete
modifier to delete rows. The steps are as follows:
- Add a
state
variable to theContentView
struct calledcountries
and initialize it with an array of country names:@State var countries = ["USA", "Canada", "England", "Cameroon", "South Africa", "Mexico" , "Japan", "South Korea"]
- Within the body variable, add a
navigationView
and aList
view that displays our array of countries. Also, include theonDelete
modifier at the end of theForEach
structure:NavigationView{ List { ForEach(countries, id: \.self) { country in Text(country) } .onDelete(perform: self.deleteItem) } .navigationBarTitle("Countries", displayMode: .inline) }
- Below the body variable's closing brace, add the
deleteItem
function:private func deleteItem(at indexSet: IndexSet){ self.countries.remove(atOffsets: indexSet) }
The resulting preview should look as follows:
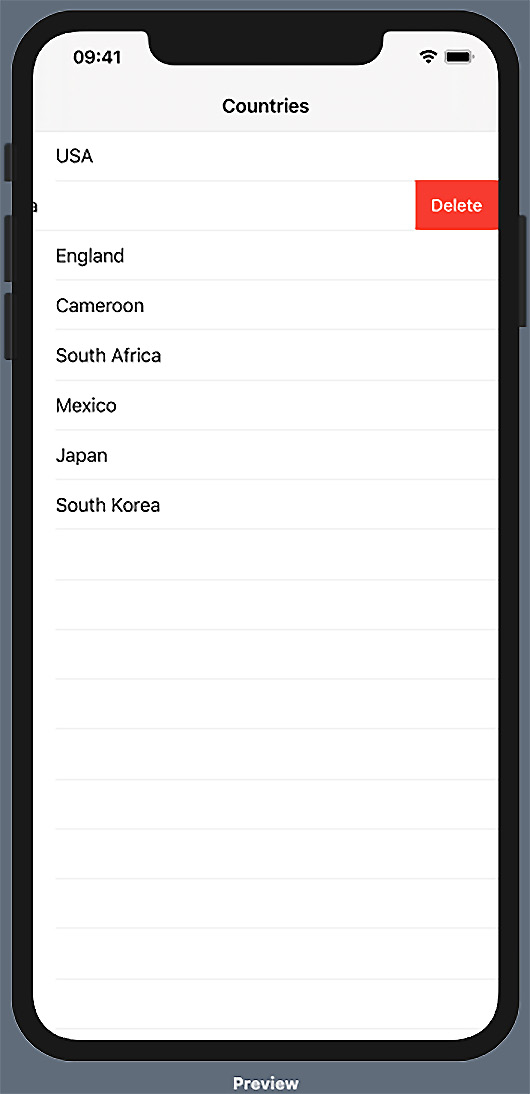
Figure 2.6 – ListRowDelete in action
Run the canvas preview and swipe right to left on a list row. The Delete button will appear and can be clicked to delete an item from the list.
How it works…
In this recipe, we introduced the .onDelete
modifier, whose perform
parameter takes a function that will be executed when clicked. In this case, deleting an item triggers the execution of our deleteItem
function.
The deleteItem
function takes a single parameter, IndexSet
, which is the index of the row to be deleted. The onDelete
modifier automatically passes the index of the item to be deleted.
There's more…
Deleting an item from a List
view can also be performed by embedding the list navigation view and adding an EditButton
component.