Text box widgets
In tkinter
, the typical textbox widget is called Entry
. In this recipe, we will add such an Entry
to our GUI. We will make our label more useful by describing what the Entry
is doing for the user.
Getting ready
This recipe builds upon the Creating buttons and changing their text property recipe.
How to do it...
# Modified Button Click Function # 1 def clickMe(): # 2 action.configure(text='Hello ' + name.get()) # Position Button in second row, second column (zero-based) action.grid(column=1, row=1) # Changing our Label # 3 ttk.Label(win, text="Enter a name:").grid(column=0, row=0) # 4 # Adding a Textbox Entry widget # 5 name = tk.StringVar() # 6 nameEntered = ttk.Entry(win, width=12, textvariable=name) # 7 nameEntered.grid(column=0, row=1) # 8
Now our GUI looks like this:
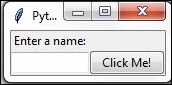
After entering some text and clicking the button, there is the following change in the GUI:
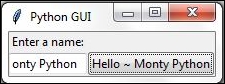
How it works...
In line 2 we are getting the value of the Entry
widget. We are not using OOP yet, so how come we can access the value of a variable that was not even declared yet?
Without using OOP classes, in Python procedural coding we have to physically place a name above a statement that tries to use that name. So how come this works (it does)?
The answer is that the button click event is a callback function, and by the time the button is clicked by a user, the variables referenced in this function are known and do exist.
Life is good.
Line 4 gives our label a more meaningful name, because now it describes the textbox below it. We moved the button down next to the label to visually associate the two. We are still using the grid layout manager, to be explained in more detail in Chapter 2, Layout Management.
Line 6 creates a variable name
. This variable is bound to the Entry
and, in our clickMe()
function, we are able to retrieve the value of the Entry
box by calling get()
on this variable. This works like a charm.
Now we see that while the button displays the entire text we entered (and more), the textbox Entry
widget did not expand. The reason for this is that we had hard-coded it to a width of 12 in line 7.
Note
Python is a dynamically-typed language and infers the type from the assignment. What this means is if we assign a string to the variable name,
the variable will be of the type string, and if we assign an integer to name,
this variable's type will be integer.
Using tkinter, we have to declare the variable name
as the type tk.StringVar()
before we can use it successfully. The reason is this that Tkinter is not Python. We can use it from Python but it is not the same language.