Time for action – transforming the viewport
Let's extend our code so that all future operations focus only on drawing within the border boundaries after the border is drawn. Use the window
and
viewport
transformation as follows:
void Widget::paintEvent(QPaintEvent *) { QPainter painter(this); painter.setRenderHint(QPainter::Antialiasing, true); QPen pen(Qt::black); pen.setWidth(4); painter.setPen(pen); QRect r = rect().adjusted(10, 10, -10, -10); painter.drawRoundedRect(r, 20, 10); painter.save(); r.adjust(2, 2, -2, -2); painter.setViewport(r); r.moveTo(0, -r.height()/2); painter.setWindow(r); drawChart(&painter, r); painter.restore(); }
Also create a protected method called drawChart()
:
void Widget::drawChart(QPainter *painter, const QRect &rect) { painter->setPen(Qt::red); painter->drawLine(0, 0, rect.width(), 0); }
Let's take a look at our output:
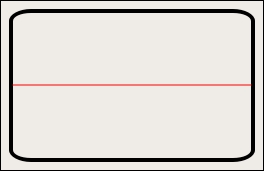
What just happened?
The first thing we did in the newly added code is call painter...