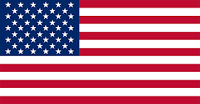

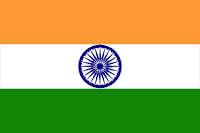
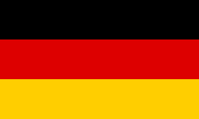
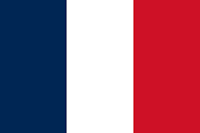

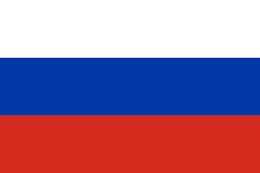
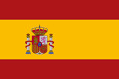



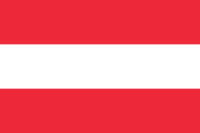

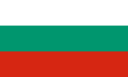
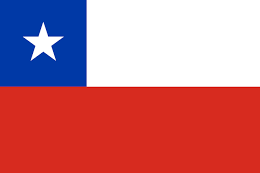

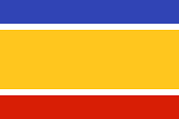
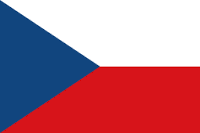
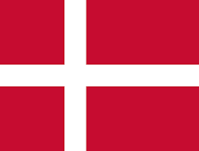
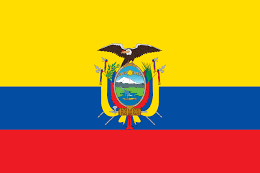
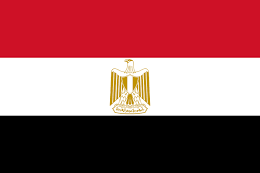

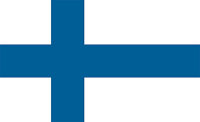
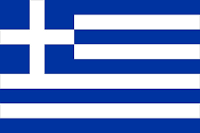
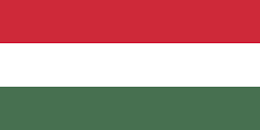
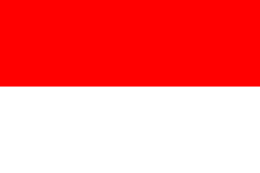

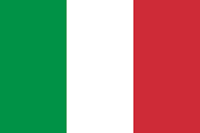

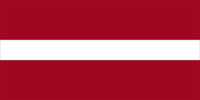
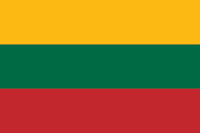
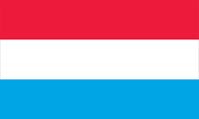
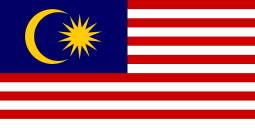

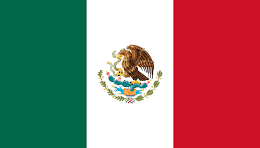

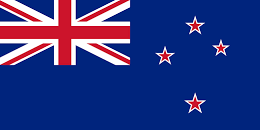



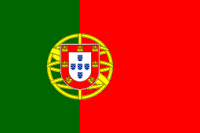
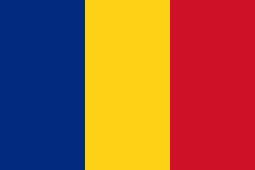


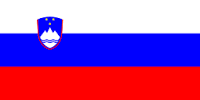
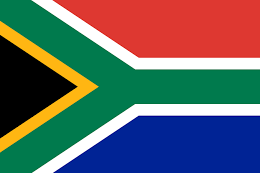

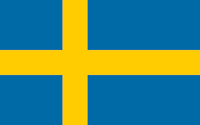

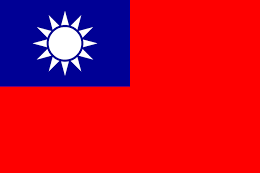
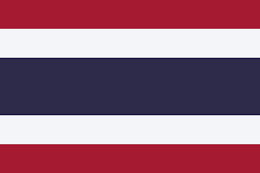
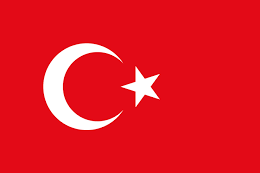
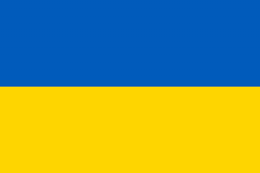
In this article by Ajitesh Kumar, the author of the book Building Web Apps with Spring 5 and Angular, we will see the key aspects of web request-response handling in relation with Spring Web MVC framework.
In this article, we will go into the details of setting up development environment for working with Spring web applications. Following are going to be the key areas we are going to look into:
(For more resources related to this topic, see here.)
Installing Java SDK
First and foremost, we will install Java SDK. We will work with Java 8 throughout this book. Go ahead and access this page (http://www.oracle.com/technetwork/java/javase /downloads/jdk8-downloads-2133151.html). Download the appropriate JDK kit. For Windows OS, there are two different versions, one for x86 and another for x64. One should select appropriate version and download “exe” file. Once downloaded, double-click on the executable file. This would start the installer. Once installed, following needs to be done:
Next, let us try and understand how to install and configure Maven, a tool for building and managing Java projects.
Installing/Configuring Maven
Maven is a tool which can be used for building and managing Java-based project. Following are some of the key benefits of using Maven as a build tool:
One can download Maven from https://maven.apache.org/download.cgi. Before installing Maven, make sure Java is installed and configured (JAVA_HOME) appropriately as mentioned in the previous section. On Windows, you could check the same by typing the command, “echo %JAVA_HOME%”:
Now, let’s look at how can we create a Java project using Maven from command prompt before we get on to creating a Maven project in Eclipse IDE. Use following mvn command to create a Java project:
mvn archetype:generate -DgroupId=com.healthapp -DartifactId=HealthApp - DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
With archetype:generate and -DarchetypeArtifactId=maven-archetypequickstart template, following project directory structure is created:
In the preceding diagram, healthapp folders within src/main and src/test folder consist of a hello world program named as "App.java" and a corresponding test program such as "AppTest.java". Also, the at the top most folder, a pom.xml file is created.
In the next section, we will install Eclipse IDE and create a maven project using the functionality provided by the IDE.
Installing Eclipse IDE
In this section, we will get ourselves setup with Eclipse IDE, a tool used by Java developers to create Java EE and web applications. Go to Eclipse website, http://www.eclipse.org and download the latest version of Eclipse and install thereafter. As we shall be working with web applications, select the option such as "Eclipse IDE for Java EE Developers" while downloading the IDE.
As you launch the IDE, it will ask to select a folder for workspace. Select appropriate path and start the IDE.
Following are some of the different types of projects developers could work using IDE:
Import existing Maven Project in Eclipse
In previous section, we have created a maven project namely HealthApp. We will now see how we can import this project into Eclipse IDE:
Figure 2: Maven project imported into Eclipse
Let's also see how one can create a new Maven project with Eclipse IDE.
Create new Maven Project in Eclipse
Follow the instructions given to create new Java Maven project with Eclipse IDE:
As a result of preceding steps, a new Maven project will be created in Eclipse. Make sure this is how it looks like: Figure 3: Maven project created within Eclipse
In next section, we will see how to install and configure Tomcat Server.
Installing/Configuring Apache Tomcat Server
In this section, we will learn about some of the following:
The Apache Tomcat software is an open source implementation of the Java Servlet, JavaServer Pages (JSPs), Java Expression Language and Java WebSocket technologies. We will work with Apache Tomcat 8.x version in this book. We will look at both Windows and Unix version of Java. One can go to http://tomcat.apache.org/ and download the appropriate version from this page. At the time of installation, it requires you to choose the path to one of the JREs installed on your computer. Once installation is complete, Apache Tomcat server is started as a Windows service. With default installation options, one can then access the Tomcat server by accessing URL such as http://127.0.0.1:8080/.
A page such as following will be displayed: Figure 4: Apache Tomcat Server Homepage
Following is how Tomcat's folder structure looks like: Figure 5: Apache Tomcat Folder Structure
In the preceding diagram, note the "webapps" folder which will contain our web apps. The following description uses the variable name such as following:
Following are most commonly used approaches to deploy web apps in Tomcat:
Let us learn how to configure Apache Tomcat from within Eclipse. This would be very useful as one could start and stop Tomcat from Eclipse while working with his/her web applications.
Adding/Configuring Apache Tomcat in Eclipse
In this section, we will learn how to add and configure Apache Tomcat in Eclipse. It would help to start and stop the server from within Eclipse IDE.
Following steps need to be taken to achieve this objective:
Installing/Configuring MySQL Database
In this section, we will learn on how to install MySQL database. Go to MySQL Downloads site (https://www.mysql.com/downloads/) and click on "Community (GPL) Downloads" under MySQL community edition. On the next page, you will see listing of several MySQL software packages. Download following:
Installing/Configuring MySQL Server
In this section, we will see how to download, install and configure the MySQL database and related utility such as MySQL Workbench. Note that MySQL Workbench is a unified visual tool which can be used by database architects, developers and DBA for activities such as data modeling, SQL development, and comprehensive administration tools for server configuration, user administration etc.
Follow the instructions given for installation & configuration of MySQL server and workbench:
Using MySQL Connector
Before testing MySQL database connection from Java program, one would need to add the MySQL JDBC connector library to the classpath. In this section, we will learn how to configure/add MySQL JDBC connector library to classpath while working with Eclipse IDE or command console.
The MySQL connector (Connector/J) comes in ZIP file (*.tar.gz). The MySQL connector is a concrete implementation of JDBC API. Once extracted, one can see a JAR file with name such as mysql-connector-java-xxx.jar. Following are different ways in which this JAR file is dealt with while working with or without IDEs such as Eclipse:
java -cp .;/path/to/mysql-connector-java-xxx.jar com.healthapp.JavaClassName
Note the "." in classpath (-cp) option. This is there to add the current directory to the classpath as well such that com.healthapp.JavaClassName can be located.
Connecting to MySQL Database from a Java Class
In this section, we will learn how to test the MySQL database connection from a Java program.
Before executing the code shown as follows in your Eclipse IDE, make sure to do the following:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
/**
* Sample program to test MySQL database connection
*/
public class App
{
public static void main( String[] args )
{
String url =
"jdbc:mysql://localhost:3306/healthapp";
String username = "root";
String password = "r00t"; //Root password set
during MySQL installation procedure as
described above.
System.out.println("Connecting database...");
try {
Connection connection =
DriverManager.getConnection(url, username,
password);
System.out.println("Database connected!");
}
catch (SQLException e) {
throw new IllegalStateException("Cannot
connect the database!", e);
}
}
}
Introduction to Dockers
Docker is a virtualization technology which helps IT organizations achieve some of the following:
In this section, we will emphasize on first point which would help us setup Spring web application development in quick and easy manner.
So far, we have seen traditional manners in which we could set up the Java web application development environment by installing different tools in independent manner and later configuring them appropriately. In a traditional setup, one would be required to setup and configure Java, Maven, Tomcat, MySQL server and so on, one tool at a time, by following manual steps. On the same lines, you could see that all of the steps described in preceding sections have to be performed one-by-one in manual fashion. Following are some of the disadvantages of setting up development/test environments in this manner:
All of the praceding disadvantages could be taken care by making use of Dockers technology. In this section, we will learn briefly about some of the following:
What are Docker Containers?
In this section, we will try and understand what are Docker containers while comparing them with real-world containers. Simply speaking, Docker is an open platform for developing, shipping and running applications. It provides the ability to package and run an application in a loosely isolated environment called a container. Before going into details of Docker containers, let us try and understand the problems that are solved by real-world containers.
What are real-world containers good for?
Following picture represents real world containers which are used to package annything and everything and, then, transport the goods from one place to other in an easy and safe manner:Figure 12: Real-world containers
The following diagram represents different form of goods which needs to be transported using different from of transport mechanisms from one place to another: Figure 13: Different forms of goods vis-a-vis different form of transport mechanisms
The following diagram displays the matrix representing need to transport each of the goods via different transport mechanism. The challenge is to make sure that these goods get transported in easy and safe manner: Figure 14: Complexity associated with transporting goods of different types using different transport mechanisms
In order to solve preceding problem of transporting the goods in safe and easy manner irrespective of transport medium, the containers are used. Look at the following diagram: Figure 15: Goods can be packed within containers, and containers can be transported.
How does Docker containers relate to the real-world containers?
Now imagine the act of moving a software application from one environment to another environment starting from development right up to production. Following diagram represents complexity associated with making different application components work in different environments: Figure 16: Complexity associated with making different application components work in different environments
As per the preceding diagram, to make different application components work in different environments (different hardware platforms), one would require to make sure environment compatible software versions and related configurations are set appropriately. Doing this using manual steps can be real cumbersome and error prone task.
This is where docker containers fit in. Following diagram represents containerizing different application components using Docker containers. As like real-world containers, it would become very easy to move the containerized application components from one environment to another with very less or no issues: Figure 17: Docker containers to move application components across different environments
Docker containers
In simple terms, Docker containers provide an isolated and secured environment for the application components to run. The isolation and security allows one or many containers to run simultaneously on a given host. Often, for simplicity sake, Docker containers are loosely termed as lightweight-VMs (Virtual Machine). However, they are very much different from the traditional VMs. Docker containers do not need hypervisors to run as like virtual machines and, thus, multiple containers can be run on a given hardware combination.
Virtual machines include the application, the necessary binaries and libraries, and an entire guest operating system; all of which can amount to tens of GBs. On the other hand, Docker Containers include the application and all of its dependencies; but share the kernel with other containers, running as isolated processes in user space on the host operating system. Docker containers are not tied to any specific infrastructure: they run on any computer, on any infrastructure, and in any cloud. This very aspect make them look like a real-world container. Following diagram sums it all: Figure 18: Difference between traditional VMs and Docker containers
Following are some of the key building blocks of Docker technology:
What are key building blocks of Dockers containers?
For setting up our development environment, we will rely on Docker containers and assemble them together using the tool called as Docker compose which we shall learn about little later. Let us understand some of the following which can also be termed as key building blocks of Docker containers:
docker build -f tomcat.df -t tomcat_debug
The preceding command would look for the Dockerfile "tomcat.df" in the current directory specified by "." and build the image with tag, "tomcat_debug".
Installing Dockers
Now that we have got an understanding on What are Dockers, lets install Dockers. We shall look into steps that are required to install Dockers on Windows OS:
Setting up Development Environment using Docker Compose
In this section, we will learn how to setup on-demand, self-service development environment using Docker compose. Following are some of the points covered in this section:
What is Docker Compose?
Docker compose is a tool for defining and running multi-container Docker applications. One will require to create a Compose file to configure the application's services. Following steps are required to be taken in order to work with Docker compose:
As we are going to setup a multi-container applications using Tomcat and MySQL as different containers, we will use Docker compose to configure both of them and, then, assemble the application.
Docker Compose script for setting up the development environment
In order to come up with a Docker compose script which can set up our Spring Web App development environment with one script execution, we will first set up images for following by creating independent Dockerfiles.
Setting up Tomcat 8.x as a Container Service
Following steps can be used to setup Tomcat 8.x along with Java 8 and Maven 3.x as one container:
FROM phusion/baseimage:0.9.17
RUN echo "deb http://archive.ubuntu.com/ubuntu trusty main
universe" > /etc/apt/sources.list
RUN apt-get -y update
RUN DEBIAN_FRONTEND=noninteractive apt-get install -y -q pythonsoftware-properties
software-properties-common
ENV JAVA_VER 8
ENV JAVA_HOME /usr/lib/jvm/java-8-oracle
RUN echo 'deb http://ppa.launchpad.net/webupd8team/java/ubuntu
trusty main' >> /etc/apt/sources.list &&
echo 'deb-src http://ppa.launchpad.net/webupd8team/java/ubuntu
trusty main' >> /etc/apt/sources.list &&
apt-key adv --keyserver keyserver.ubuntu.com --recv-keys
C2518248EEA14886 &&
apt-get update &&
echo oracle-java${JAVA_VER}-installer shared/accepted-oraclelicense-v1-1
select true | sudo /usr/bin/debconf-set-selections &&
apt-get install -y --force-yes --no-install-recommends oraclejava${JAVA_VER}-installer
oracle-java${JAVA_VER}-set-default &&
apt-get clean &&
rm -rf /var/cache/oracle-jdk${JAVA_VER}-installer
RUN update-java-alternatives -s java-8-oracle
RUN echo "export JAVA_HOME=/usr/lib/jvm/java-8-oracle" >> ~/.bashrc
RUN apt-get clean && rm -rf /var/lib/apt/lists/* /tmp/* /var/tmp/*
ENV MAVEN_VERSION 3.3.9
RUN mkdir -p /usr/share/maven
&& curl -fsSL
http://apache.osuosl.org/maven/maven-3/$MAVEN_VERSION/binaries/apac
he-maven-$MAVEN_VERSION-bin.tar.gz
| tar -xzC /usr/share/maven --strip-components=1
&& ln -s /usr/share/maven/bin/mvn /usr/bin/mvn
ENV MAVEN_HOME /usr/share/maven
VOLUME /root/.m2
RUN apt-get update &&
apt-get install -yq --no-install-recommends wget pwgen cacertificates
&&
apt-get clean &&
rm -rf /var/lib/apt/lists/*
ENV TOMCAT_MAJOR_VERSION 8
ENV TOMCAT_MINOR_VERSION 8.5.8
ENV CATALINA_HOME /tomcat
RUN wget -q
https://archive.apache.org/dist/tomcat/tomcat-${TOMCAT_MAJOR_VERSIO
N}/v${TOMCAT_MINOR_VERSION}/bin/apache-tomcat${TOMCAT_MINOR_VERSION}.tar.gz
&&
wget -qOhttps://archive.apache.org/dist/tomcat/tomcat-${TOMCAT_MAJOR_VERSIO
N}/v${TOMCAT_MINOR_VERSION}/bin/apache-tomcat${TOMCAT_MINOR_VERSION}.tar.gz.md5
| md5sum
-c - &&
tar zxf apache-tomcat-*.tar.gz &&
rm apache-tomcat-*.tar.gz &&
mv apache-tomcat* tomcat
ADD create_tomcat_admin_user.sh /create_tomcat_admin_user.sh
RUN mkdir /etc/service/tomcat
ADD run.sh /etc/service/tomcat/run
RUN chmod +x /*.sh
RUN chmod +x /etc/service/tomcat/run
EXPOSE 8080
CMD ["/sbin/my_init"]
#!/bin/bash
if [ -f /.tomcat_admin_created ]; then
echo "Tomcat 'admin' user already created"
exit 0
fi
PASS=${TOMCAT_PASS:-$(pwgen -s 12 1)}
_word=$( [ ${TOMCAT_PASS} ] && echo "preset" || echo "random" )
echo "=> Creating an admin user with a ${_word} password in Tomcat"
sed -i -r 's/</tomcat-users>//' ${CATALINA_HOME}/conf/tomcatusers.xml
echo '<role rolename="manager-gui"/>' >>
${CATALINA_HOME}/conf/tomcat-users.xml
echo '<role rolename="manager-script"/>' >>
${CATALINA_HOME}/conf/tomcat-users.xml
echo '<role rolename="manager-jmx"/>' >>
${CATALINA_HOME}/conf/tomcat-users.xml
echo '<role rolename="admin-gui"/>' >>
${CATALINA_HOME}/conf/tomcat-users.xml
echo '<role rolename="admin-script"/>' >>
${CATALINA_HOME}/conf/tomcat-users.xml
echo "<user username="admin" password="${PASS}" roles="managergui,manager-script,manager-jmx,admin-gui,
admin-script"/>" >>
${CATALINA_HOME}/conf/tomcat-users.xml
echo '</tomcat-users>' >> ${CATALINA_HOME}/conf/tomcat-users.xml
echo "=> Done!"
touch /.tomcat_admin_created
echo
"==================================================================
======"
echo "You can now configure to this Tomcat server using:"
echo ""
echo " admin:${PASS}"
echo ""
echo
"==================================================================
======"
#!/bin/bash
if [ ! -f /.tomcat_admin_created ]; then
/create_tomcat_admin_user.sh
fi
exec ${CATALINA_HOME}/bin/catalina.sh run
docker build -f tomcat.df -t demo/tomcat:8 .
docker images
docker run -ti -d -p 8080:8080 --name tomcatdev -v "$PWD":/mnt/
demo/tomcat:8
docker exec -ti tomcatdev /bin/bash
In this section, we learnt to setup Tomcat 8.5.8 along with Java 8 and Maven 3.x as one container.
Setting up MySQL as a Container Service
In this section, we will learn how to setup MySQL as a container service. In the docker terminal, execute the following command:
docker run -ti -d -p 3326:3306 --name mysqldev -e MYSQL_ROOT_PASSWORD=r00t -v "$PWD":/mnt/ mysql:5.7
The preceding command setup MySQL 5.7 version within the container and starts the mysqld service. Open MySQL Workbench and create a new connection by entering the details such as following and click "Test Connection". You should be able to establish the connection successfully: Figure 21: MySQL server running in the container and accessible from host machine at 3326 port using MySQL Workbench
Docker Compose script to setup the Dev Environment
Now, that we have setup both Tomcat and MySQL as individual containers, let us learn to create a Docker compose script using which both the containers can be started simultaneously thereby starting the Dev environment.
version: '2'
services:
web:
build:
context: .
dockerfile: tomcat.df
ports:
- "8080:8080"
volumes:
- .:/mnt/
links:
- db
db:
image: mysql:5.7
ports:
- "3326:3306"
environment:
- MYSQL_ROOT_PASSWORD=r00t
// For starting the services in the foreground
docker-compose up
// For starting the services in the background (detached mode)
docker-compose up -d
// For stopping the services
docker-compose stop
Summary
In this article, we learnt how we could start and stop the Web app Dev environment on- demand. Note that with these scripts including Dockerfiles, shell scripts and Dockercompose file, you could setup the Dev environment on any machine where Docker Toolbox could be installed.