Using a QDialog
We deserve something better than an untitled task. The user needs to define its name when it's created. The easiest path would be to display a dialog where the user can input the task name. Fortunately Qt offers us a very configurable dialog that fits perfectly in addTask()
:
#include <QInputDialog> ... void MainWindow::addTask() { bool ok; QString name = QInputDialog::getText(this, tr("Add task"), tr("Task name"), QLineEdit::Normal, tr("Untitled task"), &ok); if (ok && !name.isEmpty()) { qDebug() << "Adding new task"; Task* task = new Task(name); mTasks.append(task); ui->tasksLayout->addWidget(task); } }
The QinputDialog::getText
function is a static blocking function that displays the dialog. When the user validates/cancels the dialog, the code continues. If we run the application and try to add a new task, we'll see this:
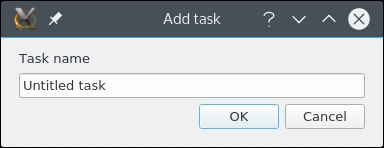
The QInputDialog::getText
signature looks like this:
QString QinputDialog::getText( QWidget* parent, const QString& title, const QString& label, QLineEdit::EchoMode mode = QLineEdit::Normal, const QString& text = QString(), bool* ok = 0, ...)
Let's break it down:
parent
: This is the parent widget (MainWindow
) to which theQinputDialog
is attached. This is another instance of theQObject
class's parenting model.title
: This is the title displayed in the window title. In our example, we usetr("Add task")
, which is how Qt handles i18n in your code. We will see later on how to provide multiple translations for a given string.label
: This is the label displayed right above the input text field.mode
: This is how the input field is rendered (password mode will hide the text).ok
: This is a pointer to a variable that is set to true if the user presses OK and to false if the user presses Cancel.QString
: The returnedQString
is what the user has typed.
There are a few more optional parameters we can safely ignore for our example.