We have already discussed the use of AsParallel() to create parallel queries. Sometimes, we may want to execute operators sequentially. We can force PLINQ to operate sequentially using the AsSequential() method. Once this method is applied to any parallel query, the following operators execute in a sequence. Consider the following code:
var range = Enumerable.Range(1, 1000);
range.AsParallel().Where(i => i % 2 == 0).AsSequential().Where(i => i % 8 == 0).AsParallel().OrderBy(i => i);
Here, the first Where class, Where(i => i % 2 == 0), will execute in parallel. The second Where class, Where(i => i % 8 == 0), however, will execute sequentially. OrderBy will also be switched to parallel execution mode.
This is shown in the following diagram:
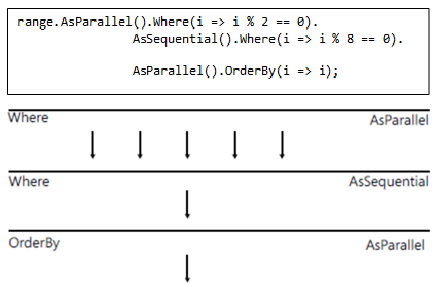
Now, we should have a good idea about how to merge synchronous and parallel LINQ...