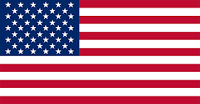

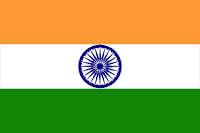
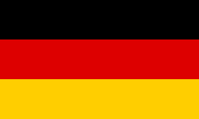
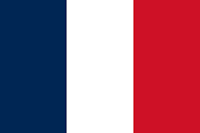

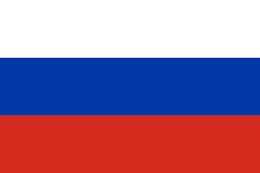
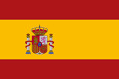



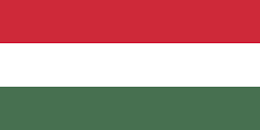
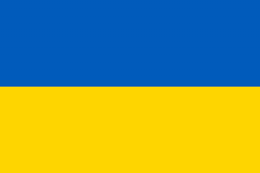
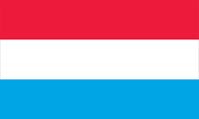

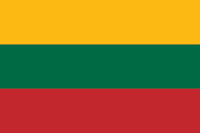

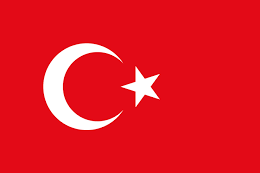


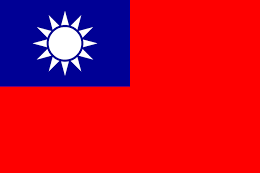
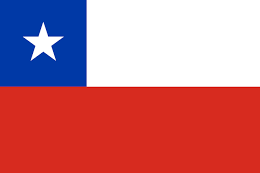

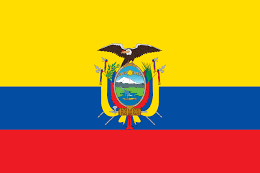
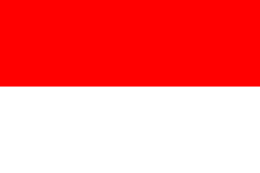
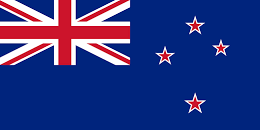
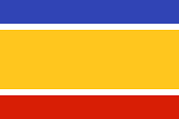
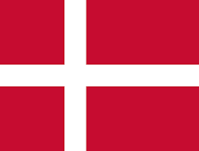
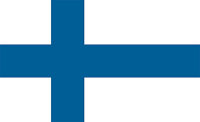


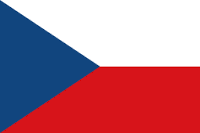
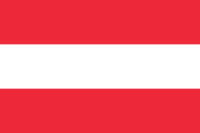
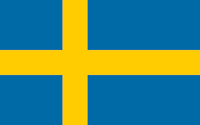
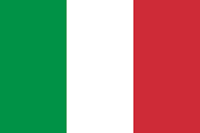
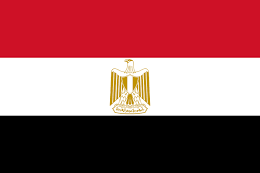

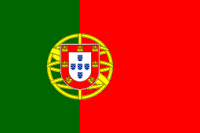
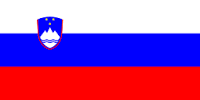

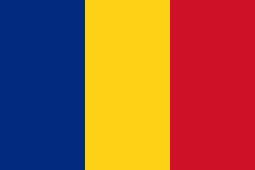
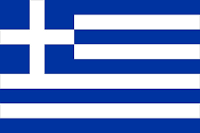


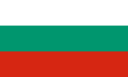
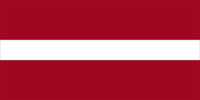
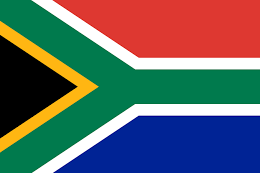
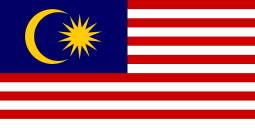



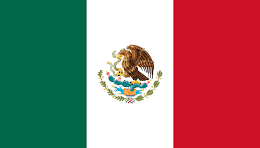
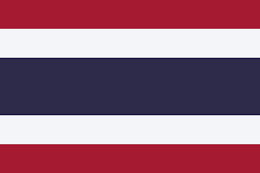
Dive deeper into the world of AI innovation and stay ahead of the AI curve! Subscribe to our AI_Distilled newsletter for the latest insights. Don't miss out – sign up today!
This article is an excerpt from the book, R Bioinformatics Cookbook - Second Edition, by Dan MacLean. Discover over 80 recipes for modeling and handling real-life biological data using modern libraries from the R ecosystem.
Embrace the power of streamlined debugging and code refinement with ChatGPT's expertise. Unravel the possibilities of effortlessly troubleshooting errors, optimizing performance, and refining code structures. This article explores how ChatGPT, armed with its extensive programming knowledge, assists in identifying and rectifying coding errors, offering tailored solutions, and fostering a deeper understanding of code logic. Dive into a journey of code enhancement, where ChatGPT becomes your indispensable companion in navigating complexities and elevating your coding experiences
ChatGPT is capable of debugging code due to its extensive training on programming-related text. It has acquired knowledge of common programming patterns and errors, allowing it to understand code snippets, identify potential issues, and provide suggestions or improvements. It can identify minor errors by highlighting syntax issues such as missing brackets, incorrect function calls, and invalid variable assignments. ChatGPT helps troubleshooting by asking clarifying questions to better understand the problem and guide developers toward the root cause, and can aid in code optimization by suggesting efficiency improvements, identifying performance bottlenecks, and proposing more optimized implementations. Lastly, the debugging process with ChatGPT can foster learning and exploration, enabling developers to discover new concepts, programming techniques, or functionalities of specific libraries or packages. In this recipe, we’ll walk through an example debugging session with ChatGPT and look at how it helps us to reflect better on our own code.
We just need an account at ChatGPT: https://chat.openai.com/.
We can debug some code as follows:
1. Set up some code that we expect to work and try it:
data <- data.frame(
predictor = c(1, 2, 3, 4, NA),
response = c("A", "B", "C", "D", "E")
)
model <- lm(response ~ predictor, data = data)
2. Send the broken code to ChatGPT:
Help me fix the following code:
data <- data.frame(
predictor = c(1, 2, 3, 4, NA),
response = c("A", "B", "C", "D", "E")
)
model <- lm(response ~ predictor, data = data)
3. Read and run the resulting response in R:
To fix the code, you need to handle the missing value (NA) in the predictor column. Here's the corrected code:
# Fixing the code
data <- data.frame(
predictor = c(1, 2, 3, 4, NA),
response = c("A", "B", "C", "D", "E")
)
# Replace NA in the predictor column with the mean value
data$predictor[is.na(data$predictor)] <- mean(data$predictor,
na.rm = TRUE)
# Convert the response variable to a factor
data$response <- as.factor(data$response)
# Perform linear regression
model <- lm(response ~ predictor, data = data)
summary(model)
In the fixed code, I replaced the NA value in the predictor column with the mean value of the remaining numeric values using the mean() function with na.rm = TRUE argument. Then, I converted the response variable to a factor using the as.factor() function. Finally, the linear regression model is computed with the corrected data, and the summary(model) displays the model summary.
4. Tell ChatGPT the code still doesn’t work:
It still doesn't work - Im getting thie error:
Error in as.character.factor(x) : malformed factor
In addition: Warning message:
In Ops.factor(r, 2) : '^' not meaningful for factors
5. Read the response and run the code:
Apologies for the oversight. The error is occurring because the
^ operator is not applicable to factors in the formula used in the linear regression. To fix this, we can convert the factor to a numeric representation using numeric codes or dummy variables. Here's the updated code:
# Fixing the code
data <- data.frame(
predictor = c(1, 2, 3, 4, NA),
response = c("A", "B", "C", "D", "E")
)
# Replace NA in the predictor column with the mean value
data$predictor[is.na(data$predictor)] <- mean(data$predictor,
na.rm = TRUE)
# Convert the response variable to a factor
data$response <- as.factor(data$response)
# Convert the factor to numeric codes
data$response <- as.numeric(data$response)
# Perform linear regression
model <- lm(response ~ predictor, data = data)
summary(model)
In the updated code, I've used as.numeric() to convert the response factor variable to numeric codes. This allows the regression model to treat it as a numerical response. Now, the lm() function should run without the error, and summary(model) will display the model summary.
6. Ask it to check whether the original code was appropriately analyzed:
Were the original data suitable for a linear model?
7. Read the response:
No, the original data provided in the code were not suitable for a linear model. Here's why:
1. The `predictor` variable was numeric, which is suitable for a linear model.
2. The `response` variable, however, was categorical with values
"A", "B", "C", "D", and "E". Categorical variables cannot be directly used as the response variable in a linear regression model because they do not represent continuous numeric values.
To use a categorical variable as the response variable in a linear model, you typically need to encode it into dummy variables or use techniques like multinomial logistic regression. Dummy variable encoding represents each category as a separate binary variable (0 or 1). Once the categorical variable is properly encoded, it can be used as the response variable in a linear regression model.
Again, that’s how the process goes though the output can be different from user to user but the meaning should be the same.
In step 1, we set up a data frame of some data as a test set to use in a linear model; we wish to examine the relationship between the two variables so assume that a linear model will work. When we run the code in R, we get this:
Error in lm.fit(x, y, offset = offset, singular.ok = singular.ok, ...) :
NA/NaN/Inf in 'y'
In addition: Warning message:
In storage.mode(v) <- "double" : NAs introduced by coercion
This is a typically confounded R error message. We want help, so in step 2, we ask ChatGPT to fix the code.
Step 3 shows us ChatGPT’s response, which suggests fixing the NA values that are in the predictor column. That seems reasonable, and, as it explains, ChatGPT gives us some code that imputes a new value from the mean of all the other values – again, a reasonable value to impute. When we run the code, it still doesn’t work and we get a new error, so in step 4, we tell ChatGPT about it and ask it to fix the new errors.
In step 5, we see an apologetic language model attempt to correct the error. It gives us a confusing reason for doing some strange text/number conversion and fixed code. When we run this new code in the console, we get output like this:
## Call:
## lm(formula = response ~ predictor, data = data)
##
## Residuals:
## 1 2 3 4 5
## -0.5 -0.5 -0.5 -0.5 2.0
##
## Coefficients:
## Estimate Std. Error t value Pr(>|t|)
## (Intercept) 0.5000 1.5546 0.322 0.769
## predictor 1.0000 0.5774 1.732 0.182
##
## Residual standard error: 1.291 on 3 degrees of freedom
## Multiple R-squared: 0.5, Adjusted R-squared: 0.3333
## F-statistic: 3 on 1 and 3 DF, p-value: 0.1817
This looks a bit strange – the residuals are weird and the rest of the values look poor. We start to question whether this was the right thing to do in the first place.
In step 6, we ask ChatGPT whether the linear model was the right sort of analysis. It responds as in step 7, telling us quite clearly that it was not appropriate.
This recipe highlights that we can use ChatGPT to fix code that doesn’t work, but shows also that ChatGPT will not reason without prompting. Here, it let us pursue a piece of code that wasn’t right for the task. As a language model, it can’t know that, even though we believe it would be evident from the question setup. It didn’t try to correct our flawed assumptions or logic. We still need to be responsible for the logic and applicability of our code.
In conclusion, ChatGPT emerges as a valuable ally in code debugging, offering insightful solutions and guiding developers toward efficient, error-free code. While it excels in identifying issues and suggesting fixes, it's crucial to recognize that ChatGPT operates within the boundaries of provided information. This journey underscores the importance of critical thinking in code analysis, reminding us that while ChatGPT empowers us with solutions, the responsibility for logical correctness and code applicability ultimately rests on the developer. Leveraging ChatGPT's expertise alongside mindful coding practices paves the way for seamless debugging and continual improvement in software development endeavors.
Professor Dan MacLean has a Ph.D. in molecular biology from the University of Cambridge and gained postdoctoral experience in genomics and bioinformatics at Stanford University in California. Dan is now Head of Bioinformatics at the world-leading Sainsbury Laboratory in Norwich, UK where he works on bioinformatics, genomics, and machine learning. He teaches undergraduates, post-graduates, and post-doctoral students in data science and computational biology. His research group has developed numerous new methods and software in R, Python, and other languages with over 100,000 downloads combined.