Spring Initializr
Do you want to auto-generate Spring Boot projects? Do you want to quickly get started with developing your application? Spring Initializr is the answer.
Spring Initializr is hosted at http://start.spring.io. The following screenshot shows how the website looks:
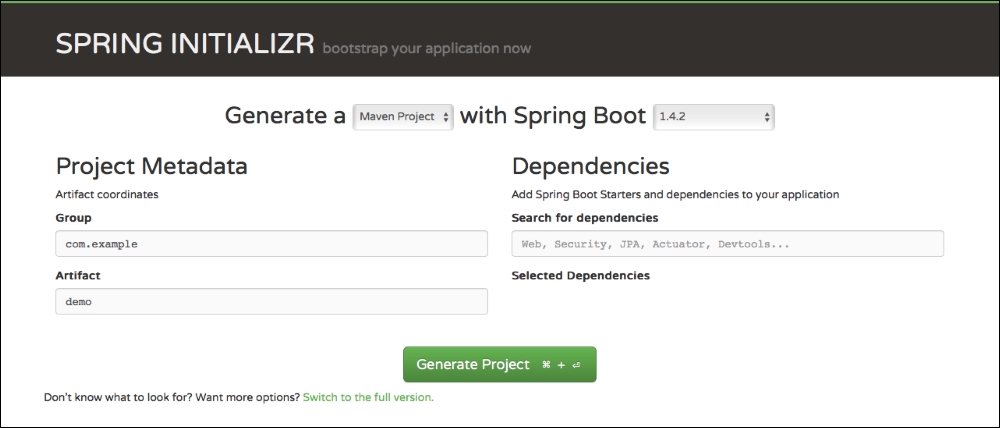
Spring Initializr provides a lot of flexibility in creating projects. You have options to do the following:
- Choose your build tool: Maven or Gradle.
- Choose the Spring Boot version you want to use.
- Configure a
Group ID
andArtifact ID
for your component. - Choose the starters (dependencies) that you would want for your project. You can click on the link at the bottom of the screen,
Switch to the full version
, to see all the starter projects you can choose from. - Choose how to package your component: JAR or WAR.
- Choose the Java version you want to use.
- Choose the JVM language you want to use.
The following screenshot shows some of the options Spring Initializr provides when you expand (click on the link) to the full version:
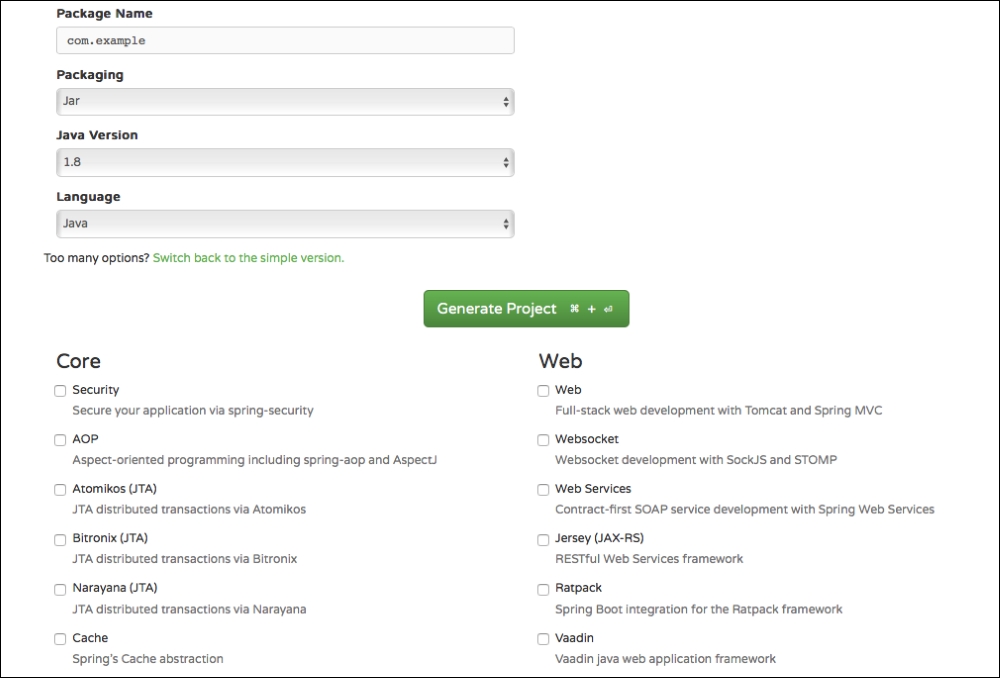
Creating Your First Spring Initializr Project
We will use the full version and enter the values, as follows:
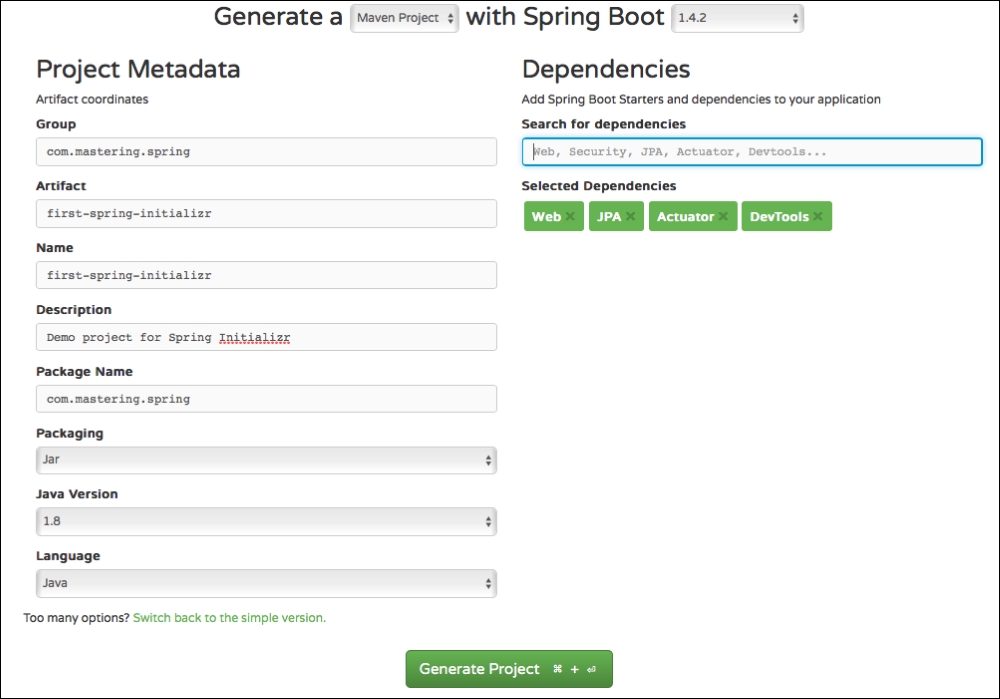
Things to note are as follows:
Build tool
:Maven
Spring Boot version
: Choose the latest availableGroup
:com.mastering.spring
Artifact
:first-spring-initializr
Selected dependencies
: ChooseWeb, JPA, Actuator and Dev Tools
. Type in each one of these in the textbox and press Enter to choose them. We will learn more about Actuator and Dev Tools in the next sectionJava version
:1.8
Go ahead and click on the Generate Project button. This will create a .zip
file and you can download it to your computer.
The following screenshot shows the structure of the project created:
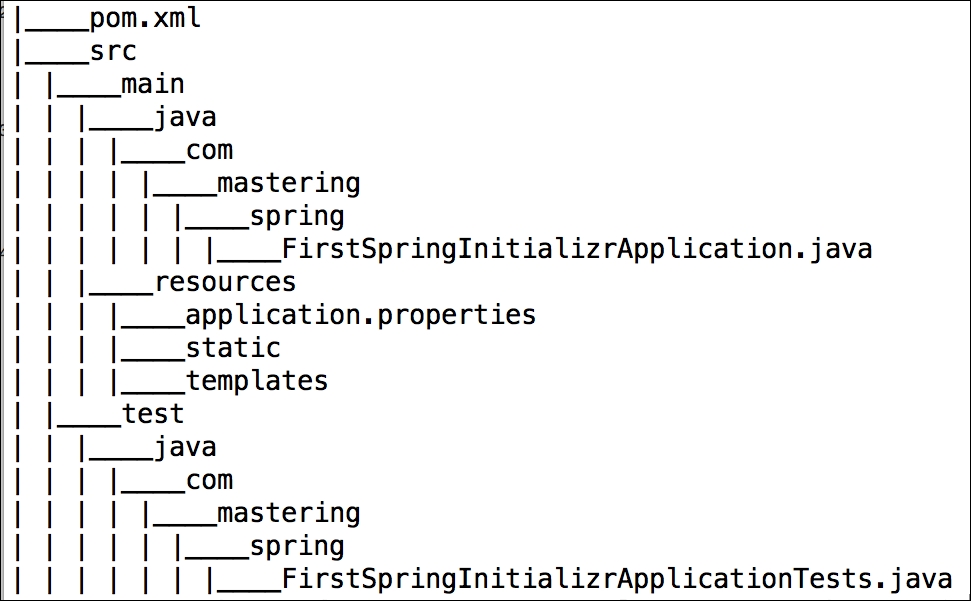
We will now import this project into your IDE. In Eclipse, you can perform the following steps:
- Launch Eclipse.
- Navigate to File | Import.
- Choose the existing Maven projects.
- Browse and select the folder that is the root of the Maven project (the one containing the
pom.xml
file). - Proceed with the defaults and click on Finish
.
This will import the project into Eclipse. The following screenshot shows the structure of the project in Eclipse:
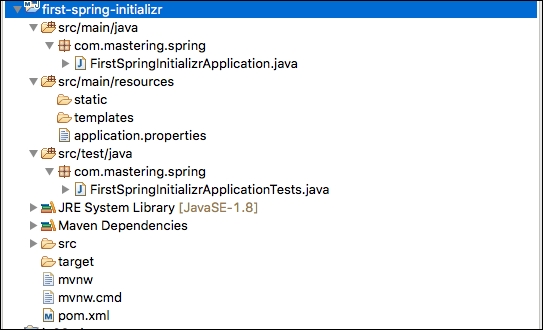
Let's look at some of the important files from the generated project.
pom.xml
The following snippet shows the dependencies that are declared:
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
A few other important observations are as follows:
- The packaging for this component is
.jar
org.springframework.boot:spring-boot-starter-parent
is declared as the parent POM<java.version>1.8</java.version>
: The Java version is 1.8- Spring Boot Maven Plugin (
org.springframework.boot:spring-boot-maven-plugin
) is configured as a plugin
FirstSpringInitializrApplication.java Class
FirstSpringInitializrApplication.java
is the launcher for Spring Boot:
package com.mastering.spring; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure .SpringBootApplication; @SpringBootApplication public class FirstSpringInitializrApplication { public static void main(String[] args) { SpringApplication.run(FirstSpringInitializrApplication.class, args); } }
FirstSpringInitializrApplicationTests Class
FirstSpringInitializrApplicationTests
contains the basic context that can be used to start writing the tests as we start developing the application:
package com.mastering.spring; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; @RunWith(SpringRunner.class) @SpringBootTest public class FirstSpringInitializrApplicationTests { @Test public void contextLoads() { } }