React 16 introduces the concept of JSX fragments. Fragments are a way to group together chunks of markup without having to add unnecessary structure to your page. For example, a common approach is to have a React component return content wrapped in a <div> element. This element serves no real purpose and adds clutter to the DOM.
Let's look at an example. Here are two versions of a component. One uses a wrapper element and one uses the new fragment feature:
import React from 'react';
import { render } from 'react-dom';
import WithoutFragments from './WithoutFragments';
import WithFragments from './WithFragments';
render(
<div>
<WithoutFragments />
<WithFragments />
</div>,
document.getElementById('root')
);
The two elements rendered are <WithoutFragments> and <WithFragments>. Here's what they look like when rendered:
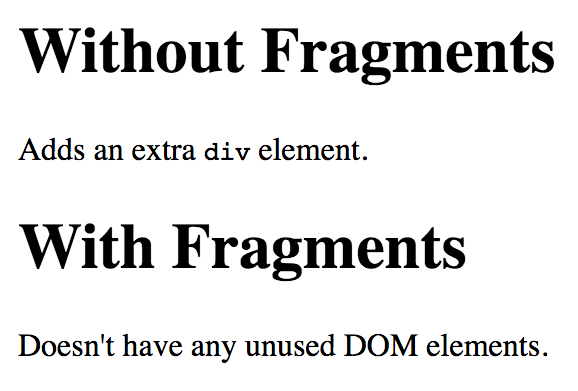
Let's compare the two approaches now.