With all the basics you have learned up to now, try to implement a URL shortening service. A URL shortener takes a very long URL and returns a shortened, crisp, and memorable URL back to the user. At first sight, it looks like magic, but it is a simple math trick.
In a single statement, URL shortening services are built upon two things:
- A string mapping algorithm to map long strings to short strings (Base 62)
- A simple web server that redirects a short URL to the original URL
There are a few obvious advantages of URL shortening:
- Users can remember the URL; easy to maintain
- Users can use the links where there are restrictions on text length, for example, Twitter
- Predictable shortened URL length
Take a look at the following diagram:
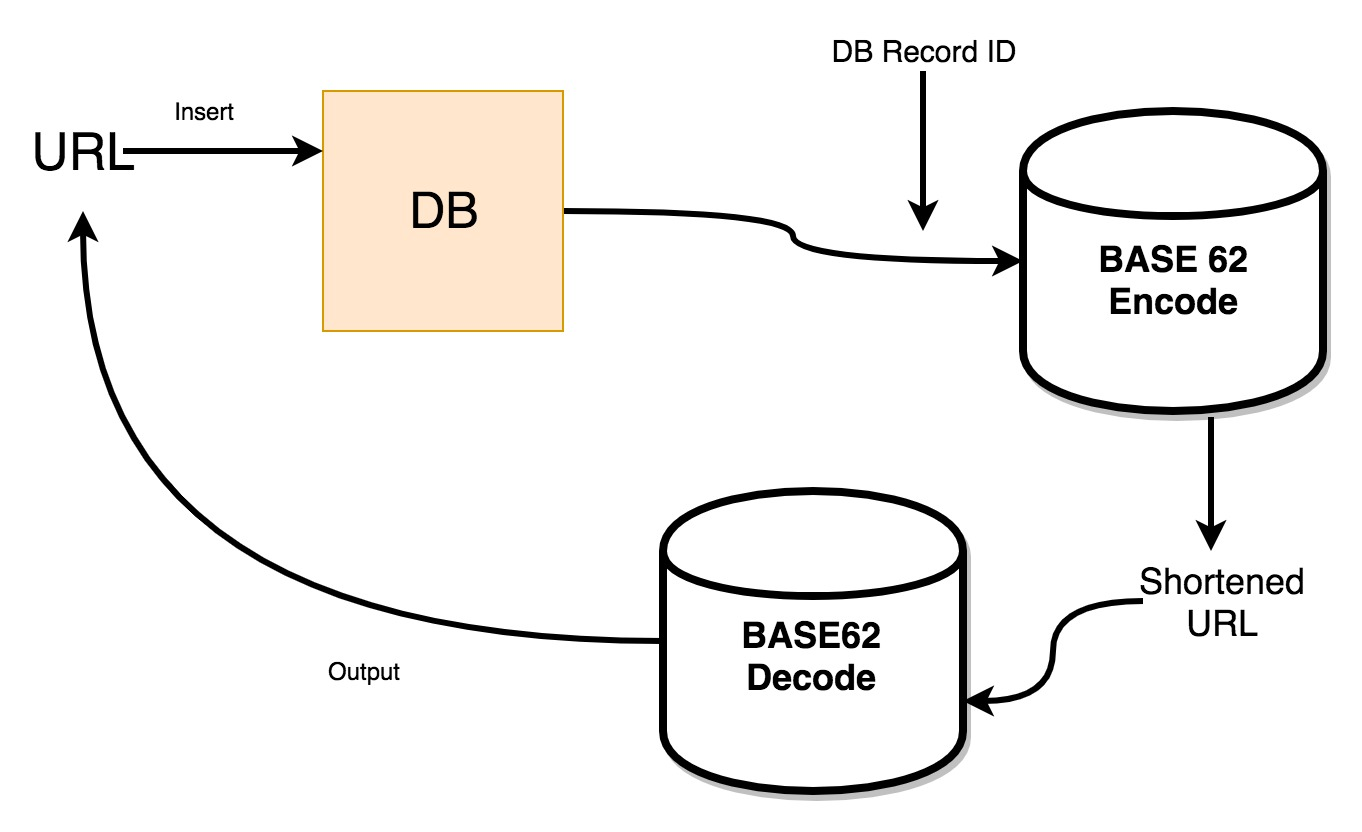
Under the hood, the following things happen in a URL shortening service:
- Take the original URL
- Apply BASE62 encoding on it; it generates a Shortened URL
- Store that URL in the database. Map it to the original URL ([shortened_url: original_url])
- Whenever a request comes to the shortened URL, just do an HTTP redirect to the original URL
We will implement a full example in upcoming chapters when we integrate databases to our API server, but before that, though, we should specify the API design documentation.
Take a look at the following table:
URL | REST Verb | Action | Success | Failure |
/api/v1/new | POST | Create a shortened URL | 200 | 500, 404 |
/api/v1/:url | GET | Redirect to original URL | 301 | 404 |
You can use a dummy JSON file/Go map to store the URL for now instead of a database.