Using external code
Package repositories, PSR standards, and social coding provide us with lots of high-quality reusable libraries and other components with free licenses. We can just install any external component in project instead of reengineering them from scratch. It improves development performance and makes for higher-quality code.
Getting ready
Create a new application by using the Composer package manager as described in the official guide at http://www.yiiframework.com/doc-2.0/guide-start-installation.html.
How to do it…
In this recipe we will try to attach some libraries manually and via Composer.
Installing a library via Composer
When you use NoSQL or other databases without autoincrement primary keys, you must generate unique identifiers manually. For example, you can use Universally Unique Identifier (UUID) instead of a numerical one. Let's do it:
- Install https://github.com/ramsey/uuid component via Composer:
composer require ramsey/uuid
- Create a demonstration console controller:
<?php namespace app\commands; use Ramsey\Uuid\Uuid; use yii\console\Controller; class UuidController extends Controller { public function actionGenerate() { $this->stdout(Uuid::uuid4()->toString() . PHP_EOL); $this->stdout(Uuid::uuid4()->toString() . PHP_EOL); $this->stdout(Uuid::uuid4()->toString() . PHP_EOL); $this->stdout(Uuid::uuid4()->toString() . PHP_EOL); $this->stdout(Uuid::uuid4()->toString() . PHP_EOL); } }
- And just run it:
./yii uuid/generate
- If successful, you'll see the following output:
25841e6c-6060-4a81-8368-4d99aa3617dd fcac910a-a9dc-4760-8528-491c17591a26 4d745da3-0a6c-47df-aee7-993a42ed915c 0f3e6da5-88f1-4385-9334-b47d1801ca0f 21a28940-c749-430d-908e-1893c52f1fe0
- That's it! Now you can use the
Ramsey\Uuid\Uuid
class in your project.
Installing libraries manually
We can install a library automatically when it is provided as a Composer package. In other cases we must install it manually.
For example, create some library examples:
- Create the
awesome/namespaced/Library.php
file with the following code:<?php namespace awesome\namespaced; class Library { public function method() { return 'I am an awesome library with namespace.'; } }
- Create the
old/OldLibrary.php
file:<?php class OldLibrary { function method() { return 'I am an old library without namespace.'; } }
- Create a set of functions as an
old/functions.php
file:<?php function simpleFunction() { return 'I am a simple function.'; }
And now set up this file in our application:
- Define the new alias for the
awesome
library namespace root in theconfig/web.php
file (inaliases
section):$config = [ 'id' => 'basic', 'basePath' => dirname(__DIR__), 'bootstrap' => ['log'], 'aliases' => [ '@awesome' => '@app/awesome', ], 'components' => [ // … ], 'params' => // … ];
or via the
setAlias
method:Yii::setAlias('@awesome', '@app/awesome');
- Define a simple class file path at the top of the
config/web.php
file:Yii::$classMap['OldLibrary'] = '@old/OldLibrary.php';
- Configure autoloading of the
functions.php
file incomposer.json
:"require-dev": { ... }, "autoload": { "files": ["old/functions.php"] }, "config": { ... },
And apply the changes:
composer update
- And now create an example controller:
<?php namespace app\controllers; use yii\base\Controller; class LibraryController extends Controller { public function actionIndex() { $awesome = new \awesome\namespaced\Library(); echo '<pre>' . $awesome->method() . '</pre>'; $old = new \OldLibrary(); echo '<pre>' . $old->method() . '</pre>'; echo '<pre>' . simpleFunction() . '</pre>'; } }
And open the page:
Using Yii2 code in other frameworks
If you want to use Yii2 framework code with other frameworks just add Yii2-specific parameters in composer.json
:
{ ... "extra": { "asset-installer-paths": { "npm-asset-library": "vendor/npm", "bower-asset-library": "vendor/bower" } } }
And install the framework:
composer require yiisoft/yii2
Now open the entry script of your application (on ZendFramework, Laravel, Symfony, and many more), require the Yii2 autoloader, and create the Yii application instance:
require(__DIR__ . '/../vendor/autoload.php'); require(__DIR__ . '/../vendor/yiisoft/yii2/Yii.php'); $config = require(__DIR__ . '/../config/yii/web.php'); new yii\web\Application($config);
That's it! Now you can use Yii::$app instances, models, widgets and other components from Yii2.
How it works…
In the first case we just install a new Composer package in our project and use it, because its composer.json
file defines all aspects of autoloading
library files.
But in the second case we did not have Composer packages and registered the files in the autoloading mechanism manually. In Yii2 we can use aliases and Yii::$classMap
for registering the roots of PSR-4 namespaces and for single files.
But as an alternative we can use Composer autoloader for all cases. Just define an extended autoload
section in the composer.json
file like this:
"autoload": { "psr-0": { "": "old/" }, "psr-4": {"awesome\\": "awesome/"}, "files": ["old/functions.php"] }
Apply the changes using this command:
composer update
Right now you can remove aliases and $classMap
definitions from your configuration files and ensure the example page still works correctly:
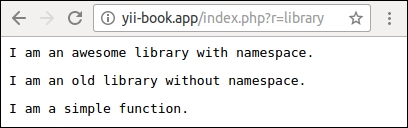
This example completely uses Composer's autoloader instead of the framework's autoloader.
See also
- For more information about integrating external code in Yii2 and framework code into our projects see the guide at http://www.yiiframework.com/doc-2.0/guide-tutorial-yii-integration.html
- For more on aliases refer to http://www.yiiframework.com/doc-2.0/guide-concept-aliases.html
- For more on the
autoload
section ofcomposer.json
refer to https://getcomposer.org/doc/01-basic-usage.md#autoloading - And also you can browse or search any Composer packages on https://packagist.org