Interfacing sensors
In this section, we are going to discuss interfacing sensors on the Pico, in particular, a reed switch. We picked a reed switch because we have all been in situations where we either cannot hear a door open, or you are not aware when someone doesn't close a door properly. We are going to discuss detecting such situations using a reed switch.
A reed switch is a type of switch that closes and makes contact in the presence of a magnetic field. Figure 3.1 shows a reed switch sold by Adafruit that could be used as a door sensor. The sensor consists of two pieces, namely, the magnet and the reed switch.
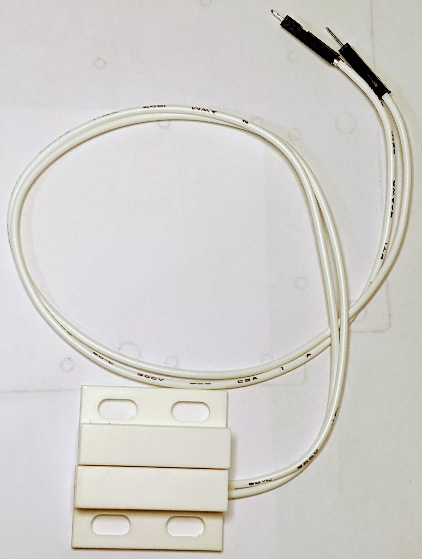
Figure 3.1 – Reed switch
The magnet is usually installed on the door while the reed switch is installed on the door frame. When the magnet is within 13 mm of the reed switch, the switch is closed. We are going to make use of this principle to detect the door state.
The steps to interface a door sensor with the Pico include the following:
- The first step is to interface the door sensor with the Pico, as shown in the following diagram:
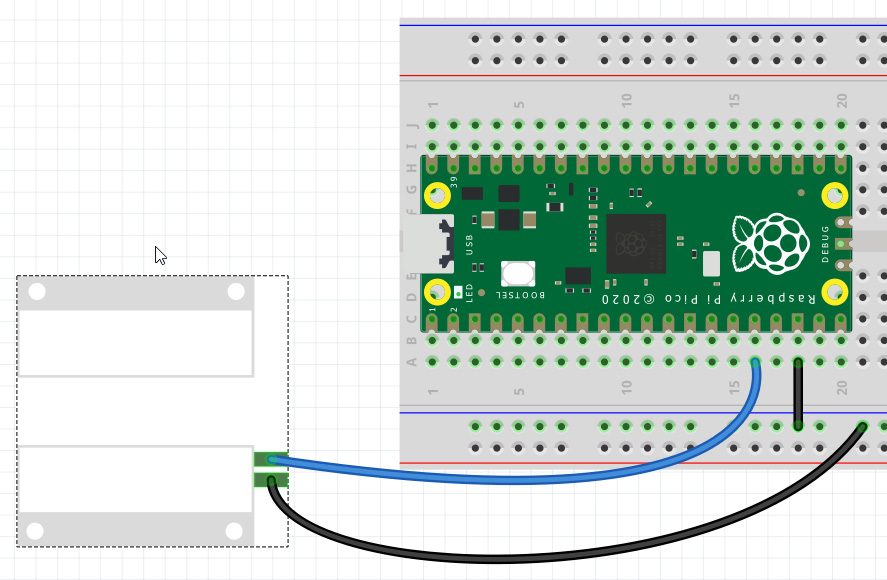
Figure 3.2 – Fritzing schematic for connecting the door sensor to the Pico
In the schematic, one wire of the reed switch is connected to the GP12 pin, while the other end is connected to the GND pin.
- We are going to discuss the code sample with the Python interpreter. The code sample discussed in this section is available for download along with this chapter as
code_door_sensor.py
. We recommend launching the Python interpreter on your Pico and working along with this example. Open the serial terminal on the Mu Integrated Development Environment (IDE) from the toolbar on the top of the IDE.

Figure 3.3 – Location of the Serial button on the toolbar
- If you see the output as shown in the following screenshot, press any key to enter the Python interpreter:
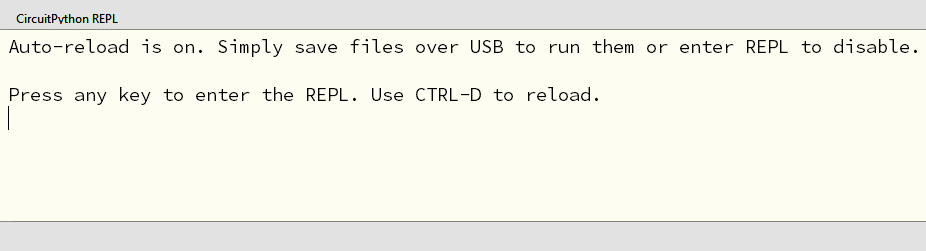
Figure 3.4 – Serial terminal on the Mu IDE
- If there is code running on the Pico, there might be a blank screen or something being printed on the serial terminal (as shown in the following screenshot). Press Ctrl + C to interrupt code execution.
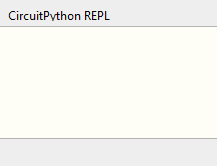
Figure 3.5 – Serial terminal with no output on the Mu IDE
- The Python interpreter would look like something similar to that shown in the following screenshot:
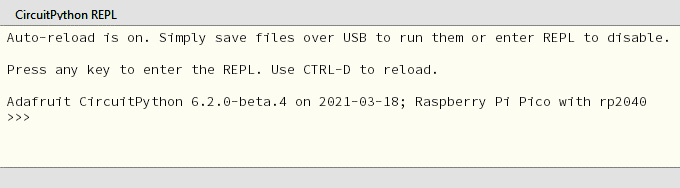
Figure 3.6 – Python interpreter on the Pico
- The first step is to import the requisite modules. For this example, we will import the
board
,time
, anddigitalio
modules:- The
board
module contains all the pin definitions for the Raspberry Pi Pico. This enables us to declare the pin we want to use in this example. - The
time
module contains the delay function. It is used to insert a deliberate delay in code execution. - The
digitalio
module is used to set up the function of a particular pin. In this example, we will be using the module to set up a pin as an input pin.
- The
The modules can be imported by running the following lines:
>>> import board >>> import digitalio >>> import time
- Since the reed switch is connected to the GP12 pin, we are going to initialize it as an input pin:
>>> switch = digitalio.DigitalInOut(board.GP12) >>> switch.direction = digitalio.Direction.INPUT
- The GP12 pin (to which we have connected the reed switch) is floating, and we need to tie it to a known state. Hence, we will use an internal pull-up resistor – a resistor. Pull-up resistors enable tying the inputs pins to a known state. This enables the prevention of any noise on the pin to be considered by the code as a change in the status of the door. In this case, we are tying pin GP12 to a high state:
>>> switch.pull = digitalio.Pull.UP
The following diagram shows the GP12 pin in a pull-up configuration:
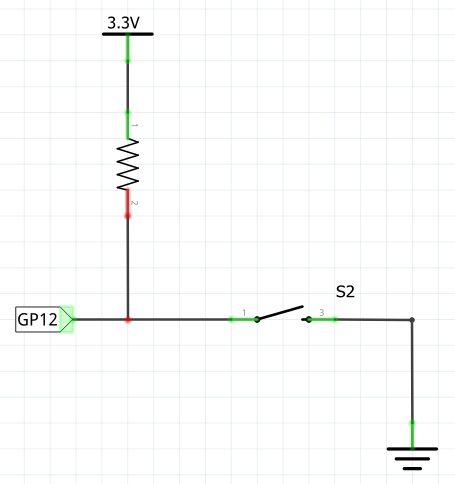
Figure 3.7 – GPIO pin pull-up configuration
- Alternatively, you could tie the GPIO pin to a pull-down state, and the GPIO pin configuration is set as follows:
>>> switch.pull = digitalio.Pull.DOWN
The following diagram shows the GP12 pin in a pull-down configuration:
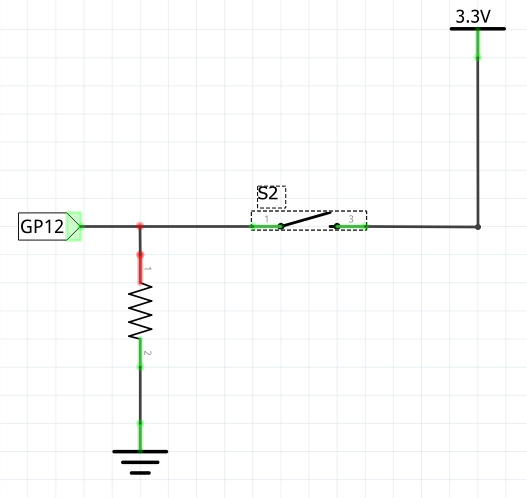
Figure 3.8 – GPIO pin pull-down configuration
- We are going to set GP12 in the pull-up state. Now, let's read the switch state in an infinite loop and test it with the door magnet as follows:
>>> while True: ... print(switch.value) ... time.sleep(1)
- Now, the terminal should start printing the door sensor's state at a 1-second interval. When the magnet is present, in other words, the door is closed, the program prints
False
to the screen. When the door is open, it printsTrue
to the screen, as can be seen on the following screen:
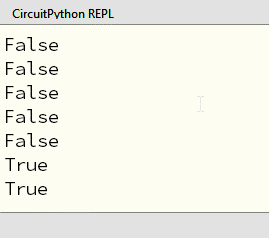
Figure 3.9 – Door sensor states printed to the screen
- Now that we have tested our code using the Python interpreter, we can save the code as
code.py
and observe the door sensor's state printed in an infinite loop:import board import digitalio import time switch = digitalio.DigitalInOut(board.GP12) switch.direction = digitalio.Direction.INPUT switch.pull = digitalio.Pull.UP while True: print(switch.value) time.sleep(1)
Have you ever wondered where such door sensors are used? Have you heard door chimes while walking into a convenience store? They use some form of door sensor. In the following photo, you can see a door sensor being used to monitor an access-controlled gate:
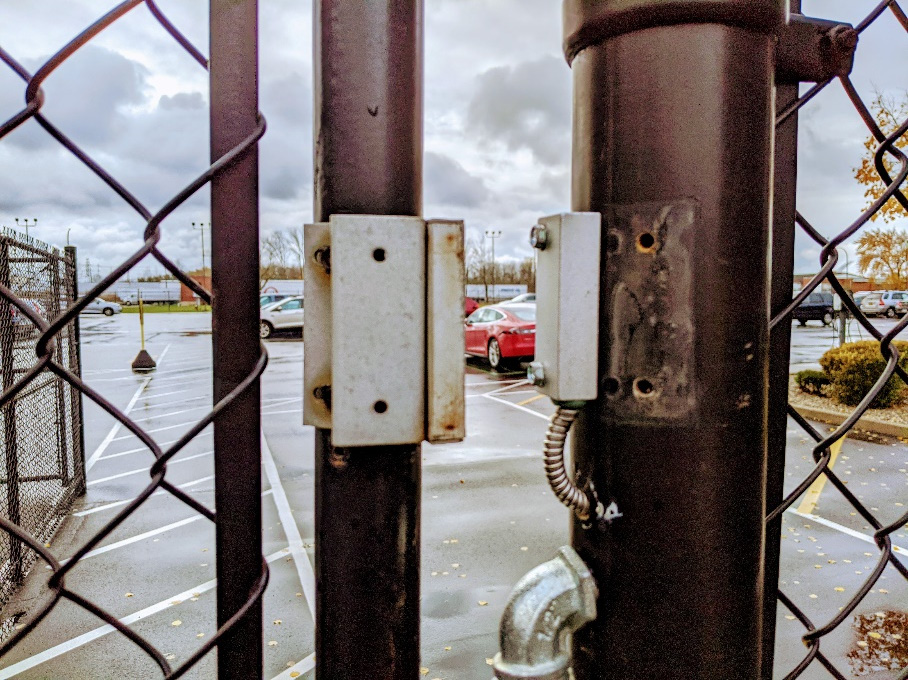
Figure 3.10 – Door sensor by an access-controlled gate
We are going to make use of this binary output from the door sensor to detect our door status and report events to the cloud or control appliances. In the next section, we are going to discuss controlling appliances inside a room based on the door sensor's state.