Implementing more complex decorators
To create more complex functions, Python allows the following kinds of commands:
@f_wrap @g_wrap def h(x): something
There's nothing in Python to stop us from stacking up decorators that modify the results of other decorators. This has a meaning somewhat like
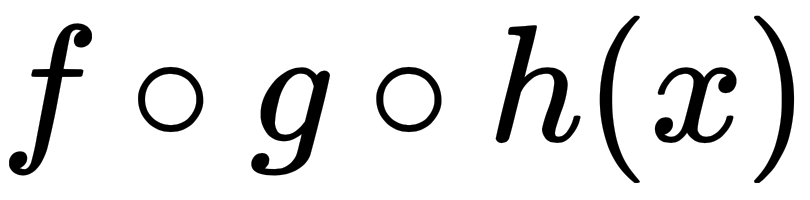
. However, the resulting name will be merely
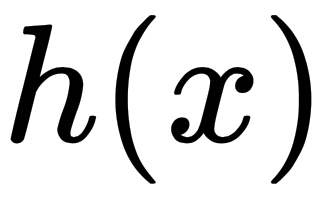
. Because of this potential confusion, we need to be cautious when creating functions that involve deeply nested decorators. If our intent is simply to handle some cross-cutting concerns, then each decorator should be designed to handle a separate concern without creating much confusion.
If, on the other hand, we're using a decoration to create a composite function, it may also be better to use the following kinds of definitions:
from typing import Callable m1: Callable[[float], float] = lambda x: x-1 p2: Callable[[float], float] = lambda y: 2**y mersenne: Callable[[float], float] = lambda x: m1(p2(x))
Each of the variables has a type hint that defines...