Responding with HTML files
Generally, when we write web servers, we do not write our HTML as strings in handlers. We write our HTML in separate files that are served by our server. We will use our application’s priv
directory to store these static files. So, let’s create a priv/static
folder in the root of our project and add an index.html
file in that folder. To add some HTML, we can use this command:
$ echo "<h1>Hello World</h1>" > priv/static/index.html
The priv directory in OTP
In OTP (Open Telecom Platform or Erlang) and Elixir, the priv
directory is a directory specific to an application that is intended to store files needed by the application when it is running. Phoenix, for example, uses the priv/static
directory to store processed JavaScript and CSS assets for runtime usage.
Let’s add an endpoint to our server that returns a static HTML file:
lib/cowboy_example/router.ex
defmodule CowboyExample.Router do @moduledoc """ This module defines routes and handlers for the web server """ alias CowboyExample.Router.Handlers.{Root, Greet, Static} @doc """ Returns the list of routes configured by this web server """ def routes do [ {:_, [ {"/", Root, []}, {"/greet/:who", [who: :nonempty], Greet, []}, # Add this line {"/static/:page", [page: :nonempty], Static, []} ]} ] end end
Now, we need a static handler module, which will look for and respond with the given page in the /priv/static
folder and, if not found, will return a 404
error:
lib/cowboy_example/router/handlers/static.ex
defmodule CowboyExample.Router.Handlers.Static do @moduledoc """ This module defines the handler for "/static/:page" route. """ require Logger @doc """ This handles "/static/:page" route, logs the requests and responds with the requested static HTML page. Responds with 404 if the page isn't found in the priv/static folder. """ def init(req0, state) do Logger.info("Received request: #{inspect req0}") page = :cowboy_req.binding(:page, req0) req1 = case html_for(page) do {:ok, static_html} -> :cowboy_req.reply( 200, %{"content-type" => "text/html"}, static_html, req0 ) _ -> :cowboy_req.reply( 404, %{"content-type" => "text/html"}, "404 Not found", req0 ) end {:ok, req1, state} end defp html_for(page) do priv_dir = :cowboy_example |> :code.priv_dir() |> to_string() page_path = priv_dir <> "/static/#{page}" File.read(page_path) end end
In the preceding module, the html_for/1
function is responsible for fetching the HTML files from our application’s priv
directory, for a given path. If the file is present, the function returns {:ok, <file_contents>}, >}
; otherwise, it returns an error, upon which we will respond with a 404
message.
We can test the preceding route by restarting our server again and making a request to the /static/index.html
path. But this time, let us use the web browser in order to render the HTML contents properly. Here’s what you should see:
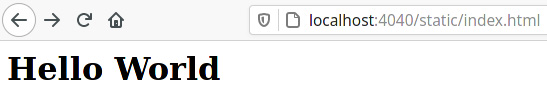
Figure 1.2 – Successful HTML response
Also, to make sure our 404
handler is working correctly, let’s make a browser request to /static/bad.html
, a file not present in our application’s priv
directory. You should see a 404
message:

Figure 1.3 – Failed HTML response
Now, we have a web server that can respond with static HTML files. It’s time to see how we can go about testing it.