Previous recipes cover how to work with text inputs; we may want to enforce some inputs to contain only numerical values. This is the use case for the Spinbox and Scale classes—both widgets allow users to select a numerical value from a range or a list of valid options, but there are several differences in the way they are displayed and configured.
Selecting numerical values
How to do it...
This program has Spinbox and Scale for selecting an integer value from 0 to 5:
import tkinter as tk class App(tk.Tk): def __init__(self): super().__init__() self.spinbox = tk.Spinbox(self, from_=0, to=5) self.scale = tk.Scale(self, from_=0, to=5, orient=tk.HORIZONTAL) self.btn = tk.Button(self, text="Print values", command=self.print_values) self.spinbox.pack() self.scale.pack() self.btn.pack() def print_values(self): print("Spinbox: {}".format(self.spinbox.get())) print("Scale: {}".format(self.scale.get())) if __name__ == "__main__": app = App() app.mainloop()
In the preceding code, for debugging purposes, we added a button that prints the value of each widget when you click on it:
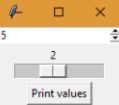
How it works...
Both classes accept the from_ and to options to indicate the range of valid values—the trailing underscore is necessary because the from option was originally defined in Tcl/Tk, but it is a reserved keyword in Python.
A handy functionality of the Scale class is the resolution option, which sets the precision of the rounding. For instance, a resolution of 0.2 will allow the user to select the values 0.0, 0.2, 0.4, and so on. The value of this option is 1 by default, so the widget rounds all values to the nearest integer.
As usual, the value of each widget can be retrieved with the get() method. An important difference is that Spinbox returns the number as a string, whereas Scale returns an integer value or a float value if the rounding accepts decimal values.
There's more...
The Spinbox class has a similar configuration to the Entry widget, such as the textvariable and validate options. You can apply all these patterns to spinboxes with the main difference that it restricts to numerical values.
See also
- The Tracing text changes recipe