2. Exemplifying the usage of text block delimiters
Remember from the previous problem, Creating a multiline SQL, JSON, and HTML string, that a text block is syntactically delimited by an opening and a closing delimiter, represented by three double quotation marks, """
.
The best approach for exemplifying the usage of these delimiters consists of three simple steps: consider an example, inspect the output, and provide a conclusion. This being said, let’s start with an example that imitates some of the JEP’s examples:
String sql= """
UPDATE "public"."office"
SET ("address_first", "address_second", "phone") =
(SELECT "public"."employee"."first_name",
"public"."employee"."last_name", ?
FROM "public"."employee"
WHERE "public"."employee"."job_title" = ?)""";
So by following the JEP examples, we have to align the content with the opening delimiter. It’s probable that this alignment style is not consistent with the rest of our code and is not such a good practice. What will happen with the text block content if we rename the sql
variable updateSql
, updateOfficeByEmployeeJobTitle
, or something else? Obviously, in order to preserve the alignment, this will push our content to the right even more. Fortunately, we can shift-left the content without affecting the final result, as follows:
String sql = """
UPDATE "public"."office"
SET ("address_first", "address_second", "phone") =
(SELECT "public"."employee"."first_name",
"public"."employee"."last_name", ?
FROM "public"."employee"
WHERE "public"."employee"."job_title" = ?""";
Shifting right the opening/closing delimiters themselves will not affect the resulting String
. It is unlikely that you’ll have a good reason to do this, but just for the sake of completion, the following example produces the same result as the previous two examples:
String sql = """
UPDATE "public"."office"
SET ("address_first", "address_second", "phone") =
(SELECT "public"."employee"."first_name",
"public"."employee"."last_name", ?
FROM "public"."employee"
WHERE "public"."employee"."job_title" = ? """;
Now, let’s see something more interesting. The opening delimiter doesn’t accept content on the same line, while the closing delimiter sits to the right at the end of the content. However, what happens if we move the closing delimiter to its own line, as in the following two examples?
String sql= """
UPDATE "public"."office"
SET ("address_first", "address_second", "phone") =
(SELECT "public"."employee"."first_name",
"public"."employee"."last_name", ?
FROM "public"."employee"
WHERE "public"."employee"."job_title" = ?
""";
String sql= """
UPDATE "public"."office"
SET ("address_first", "address_second", "phone") =
(SELECT "public"."employee"."first_name",
"public"."employee"."last_name", ?
FROM "public"."employee"
WHERE "public"."employee"."job_title" = ?
""";
This time, the resulting string contains a new line at the end of the content. Check the following figure (the text -- BEFORE TEXT BLOCK –
and -- AFTER TEXT BLOCK --
are just guidelines added via System.out.println()
to help you delimit the text block itself; they are not necessary and not part of the text block):
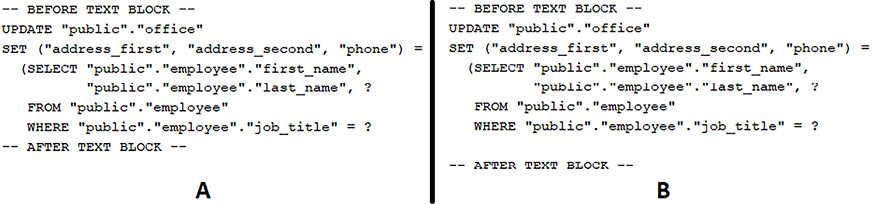
Figure 1.1: Move the closing delimiter to its own line, vertically aligned with the opening delimiter
In the left figure (A) the closing delimiter is at the end of the content. However, in the right figure (B), we moved the closing delimiter to its own line, and as you can see, the resulting String
was enriched with a new line at the end.
Important note
Placing the closing delimiter on its own line will append a new line to the final String
. Also, pay attention that vertically aligning the opening delimiter, the content, and the closing delimiter to the left margin may result later in extra work. If the variable name is modified, then manual re-indentation is needed to maintain this alignment.
So pay attention to how you place the closing delimiter.
Do you find this weird? Well, that’s not all! In the previous example, the closing delimiter was placed on its own line but vertically aligned with the opening delimiter. Let’s take a step forward and let’s shift-left the end delimiter, as in the following example:
String sql= """
UPDATE "public"."office"
SET ("address_first", "address_second", "phone") =
(SELECT "public"."employee"."first_name",
"public"."employee"."last_name", ?
FROM "public"."employee"
WHERE "public"."employee"."job_title" = ?
""";
The following figure reveals the effect of this action:
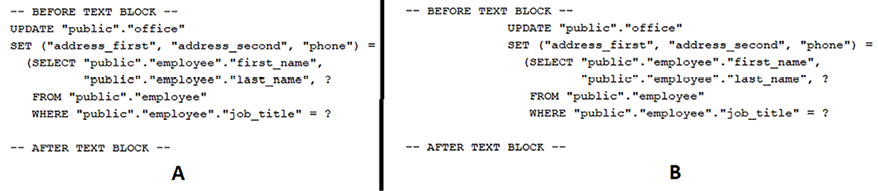
Figure 1.2: Moving the closing delimiter to its own line and shifting it to the left
In the left figure (A), we have the closing delimiter on its own line and aligned with the opening delimiter. In the right figure (B), we have the effect of the previous code. Moving the closing delimiter to the left results in an additional indentation of the content to the right. The additional indentation depends on how much we shift-left the closing delimiter.
Important note
Placing the closing delimiter on its own line and shifting it to the left will append a new line and additional indentation to the final String
.
On the other hand, if we move the closing delimiter to its own line and shift it to the right, it doesn’t affect the final String
:
String sql= """
UPDATE "public"."office"
SET ("address_first", "address_second", "phone") =
(SELECT "public"."employee"."first_name",
"public"."employee"."last_name", ?
FROM "public"."employee"
WHERE "public"."employee"."job_title" = ?
""";
This code appends a new line to the final String
but doesn’t affect indentation. In order to better understand the behavior of opening/closing delimiters, you have to explore the next problem.