Getting it rolling
OK, that's a lot of theory, so now let's fire up one of our motors via Raspberry Pi. To do that, go ahead and connect the motor and the motor driver as shown:
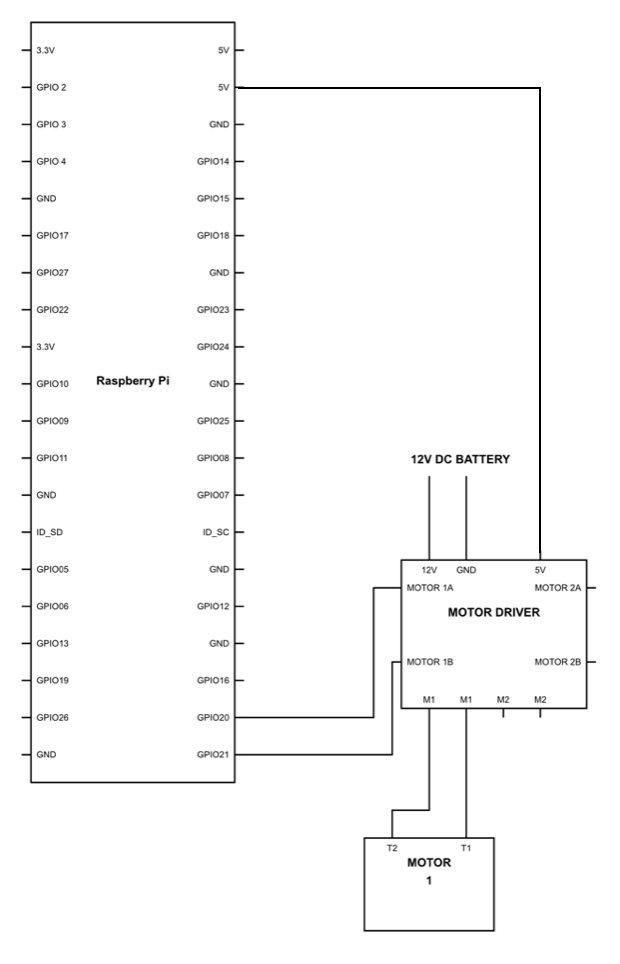
Now, once you are done with it, let's upload the code and see what happens:
import RPi.GPIO as GPIO from time import sleep GPIO.setmode(GPIO.BCM) Motor1R = 20 Motor1L = 21 GPIO.setup(Motor1R,GPIO.OUT) GPIO.setup(Motor1L,GPIO.OUT) GPIO.output(Motor1R,GPIO.HIGH) GPIO.output(Motor1L,GPIO.LOW) sleep(5) GPIO.output(Motor1R,GPIO.LOW) GPIO.output(Motor1L,GPIO.HIGH) sleep(5) GPIO.cleanup()
Now, let's understand the code a bit:
Motor1R = 20 Motor1L = 21
Pin number 20
is connected to IN 1 of the motor driver. For convenience, we have changed motor 1 right to Motor1R
; in reality, the motor can spin in any direction but we have just written this for convenience and understanding. Similarly, we have done this for Motor1L
as well. This is connected to IN 2, hence this will lead to the motor spinning in the other direction:
GPIO...