Ray
A ray is represented by a point in space and a direction. The ray extends from the point to infinity in the given direction. For our purposes, the direction of a ray is always assumed to be normalized:
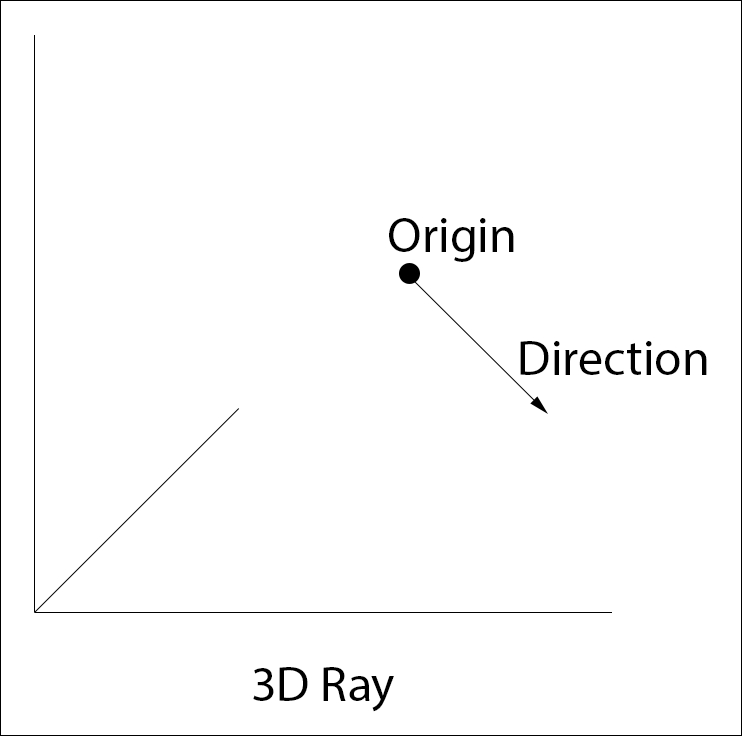
Getting ready
We are going to declare a new Ray
structure. This new structure will consist of a Point
representing the origin of the ray and a vec3
representing the direction of the ray. It is assumed that the direction vector will always be normalized. We will also implement a helper function to create a ray given two points.
How to do it…
Follow these steps to implement a 3D ray:
Declare the new
Ray
structure inGeometry3D.h
:typedef struct Ray { Point origin; vec3 direction; inline Ray() : direction(0.0f, 0.0f, 1.0f) {} inline Ray(const Point& o, const vec3& d) : origin(o), direction(d) { NormalizeDirection(); } inline void NormalizeDirection() { Normalize(direction); } } Ray;
Declare the
FromPoints
helper function inGeometry3D.h
...