Pandas Series
A Pandas Series is a one‐dimensional NumPy‐like array, with each element having an index (0, 1, 2, … by default); a Series behaves very much like a dictionary that includes an index. Figure 3.1 shows the structure of a Series in Pandas.
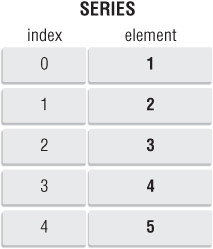
Figure 3.1: A Pandas Series
To create a Series, you first need to import the pandas
library (the convention is to use pd
as the alias) and then use the Series
class:
import pandas as pd
series = pd.Series([1,2,3,4,5])
print(series)
The preceding code snippet will print the following output:
0 1
1 2
2 3
3 4
4 5
dtype: int64
By default, the index of a Series starts from 0.
Creating a Series Using a Specified Index
You can specify an optional index for a Series using the index
parameter:
series = pd.Series([1,2,3,4,5], index=['a','b','c','d','c'])
print(series)
The preceding code snippet prints out the following:
a 1
b 2
c 3
d 4
c 5
dtype...