Using XMLSerializer to control message serialization
By default, WCF runtime uses DataContractSerializer
to perform data serialization and deserialization. However, in some cases, we will prefer using XMLSerializer
, which will give developers more control over the serialized XML content or will work more closely with some POX clients (like ASMX Web Service client).
How to do it...
First, we should make our data type ready for
XMLSerializer
. This can be done by adding XML serialization attributes on our data types. The followingUser
class has been decorated with several XML serialization attributes (XmlRootAttribute
for top-level type andXmlElementAttribute
for type members).[XmlRoot(ElementName="UserObject",Namespace="http://wcftest.org/xmlserializer")] public class User { [XmlElement(ElementName="FName")] public string FirstName { get; set; } [XmlElement(ElementName = "LName")] public string LastName { get; set; } [XmlElement(ElementName = "IsEnabled")] public bool Enabled { get; set; } }
Then, we need to apply
XmlSerializerFormatAttribute
on theServiceContract
type used in our service (see theITestService
interface shown as follows):[ServiceContract] [XmlSerializerFormat(Style=OperationFormatStyle.Document)] public interface ITestService { [OperationContract] void SendUser(User user); }
How it works...
When we apply the XmlSerializerFormatAttribute on the ServiceContract, the WCF runtime will use XMLSerializer
as the default Serializer to serialize data and deserialize SOAP messages. Also, the auto-generated service metadata will output the data type schema based on the class's XML serialization attributes. For the User
class mentioned in the previous code example, service metadata will use the schema as shown in the next screenshot to represent its XML format:
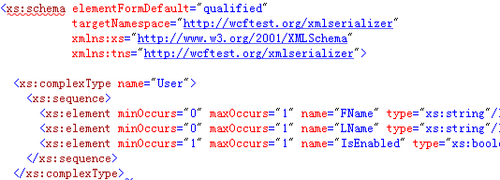
By capturing the underlying SOAP message, we can find that the XML content of the serialized User
object conforms to the metadata schema defined earlier, which is controlled by those XML serialization attributes applied on the user
class (refer to the next screenshot):
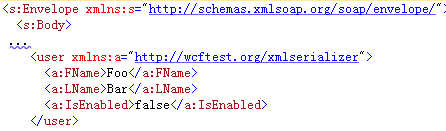
See also
Complete source code for this recipe can be found in the
\Chapter 1\recipe4\
folder