Multidimensional subscripts
While the most common subscripts are the ones that take a single parameter, subscripts are not limited to single parameters. They can take any number of input parameters, and these parameters can be of any type.
Let's see how we could use a multidimensional subscript to implement a Tic-Tac-Toe board. A Tic-Tac-Toe board looks similar to this:
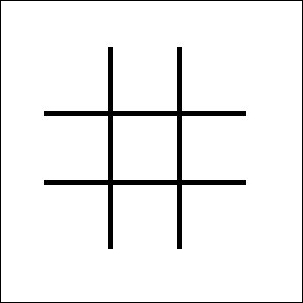
The board can be represented by a two-dimensional array where each dimension has three elements. Each player will then take a turn placing his/her pieces (typically x or o) onto the board until one player has three pieces in a row or the board is full.
Let's see how we could implement a Tic-Tac-Toe board using a multidimensional array and multidimensional subscripts:
struct TicTacToe { var board = [["","",""],["","",""],["","",""]] subscript(x: Int, y: Int) -> String { get { return board[x][y] } set { board[x][y] = newValue } } ...