Mesh object
A mesh is just a large collection of triangles:
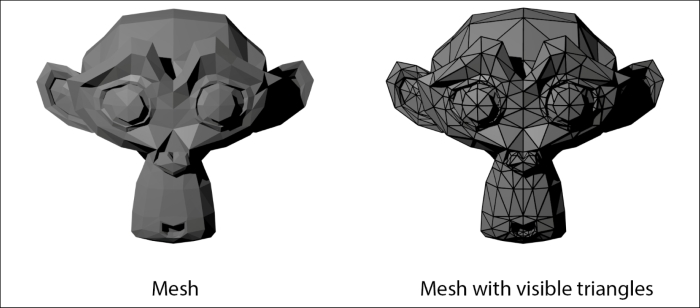
For collision detection, a mesh should be treated as a linear list of triangles. Meshes can be constructed by hand, or loaded from a file. An OBJ
loader sample is included with the code accompanying this chapter.
Getting ready
In this section, we are going to declare the Mesh
structure that will be used to test for collisions against arbitrary 3D models.
How to do it…
Follow these steps to implement a mesh primitive:
- Declare the
Mesh
structure inGeometry3D.h
:typedef struct Mesh {
- We need to know how many triangles the mesh will have:
int numTriangles;
- With this anonymous union we can access the data of the triangle in one of three ways. We can access it as triangle primitives, as points of a triangle or the ray float components:
union { Triangle* triangles;//size = numTriangles Point* vertices; //size = numTriangles * 3 float* values; //size = numTriangles * 3 * 3 }; } Mesh;