This is another minor improvement to the C# language, but one that I'm sure will often be used by developers. One of my first jobs as a young man was working for a logistics company. These folks used to supply parts to Volkswagen, and the most critical parts were flown in by air freight from Germany or elsewhere. I will never forget the 9- and 12-digit shipping numbers the logistics people used to throw around in casual conversation. I wondered how they were able to remember literally hundreds of varying shipping numbers during the course of a year. After listening to them for a while, I noticed that they were saying these numbers with slight pauses after every third number. Even just looking at the 12-digit number 395024102833 is visually taxing. Imagine doing this several times a day, including memorizing the fast movers on the next shipment (I'm not even going to go into the printed shipment manifest, which was a nightmare). It is, therefore, easier to think of the number as 395-024-102-833 and this makes it easier to spot patterns. This is essentially exactly what C# 7.0 now allows developers to do with literals.
Improvements to literals
Getting ready
Number literals can sometimes be difficult to read. This is why C# 7.0 introduces the underscore (_) to act as a digit separator inside of number literals. C# 7.0 also introduces binary literals, which allow you to specify bit patterns directly without needing to know hexadecimal.
How to do it...
- Add the following lines of code to your project. It is clear that the newNum literal is easier to read, especially if you read it in groups of three.
var oldNum = 342057239127493;
var newNum = 342_057_239_127_493;
WriteLine($"oldNum = {oldNum} and newNum = {newNum}");
- If you run the console application, you will see that the values of the two number literals are exactly the same:
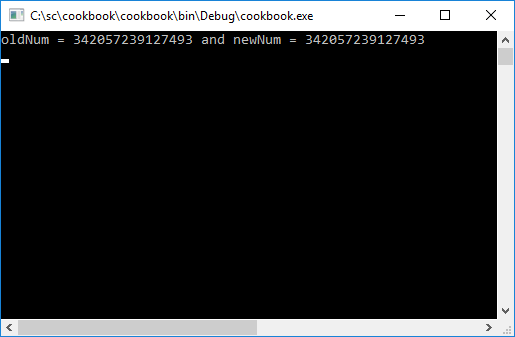
- The same logic is true for binary literals. You can now express them as follows:
var binLit = 0b1010_1100_0011_0010_0001_0000;
How it works...
This is merely syntactical sugar for literals. I'm sure that there is much more to it going on in the background, but the implementation of this in your code is really straightforward.