TensorFlow code structure
The TensorFlow programming model signifies how to structure your predictive models. A TensorFlow program is generally divided into four phases when you have imported the TensorFlow library:
- Construction of the computational graph that involves some operations on tensors (we will see what a tensor is soon)
- Creation of a session
- Running a session; performed for the operations defined in the graph
- Computation for data collection and analysis
These main phases define the programming model in TensorFlow. Consider the following example, in which we want to multiply two numbers:
import tensorflow as tf # Import TensorFlow x = tf.constant(8) # X op y = tf.constant(9) # Y op z = tf.multiply(x, y) # New op Z sess = tf.Session() # Create TensorFlow session out_z = sess.run(z) # execute Z op sess.close() # Close TensorFlow session print('The multiplication of x and y: %d' % out_z)# print result
The preceding code segment can be represented by the following figure:
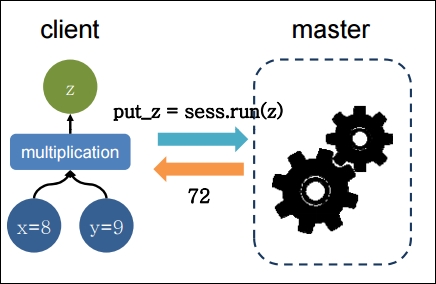
Figure...