Scala pattern matching
Scala has very useful, built-in pattern matching. Pattern matching can be used to test for exact and/or partial matches of entire values, parts of objects, you name it.
We can use this sample script for reference:
def matchTest(x: Any): Any = x match { case 7 => "seven" case "two" => 2 case _ => "something" } val isItTwo = matchTest("two") val isItTest = matchTest("test") val isItSeven = matchTest(7)
We define a function called matchTest
. The matchTest
 function takes any kind of argument and can return any type of result. (Not sure if that is real-life programming).
The keyword of interest is match
. This means the function will walk down the list of choices until it gets a match on to the x
 value passed and then returns.
As you can see, we have numbers and strings as input and output.
The last case
 statement is a wildcard, and _
 is catchall
, meaning that if the code gets that far, it will match any argument.
Â
We can see the output as follows:
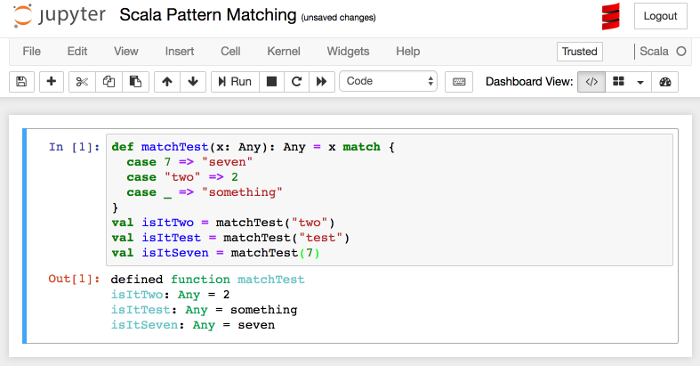