Generating random numbers
Sometimes you need to generate random numbers, perhaps in a game that simulates rolls of a die, or for use with cryptography in encryption or signing.
There are a couple of classes that can generate random numbers in .NET Standard, as shown in the following diagram:
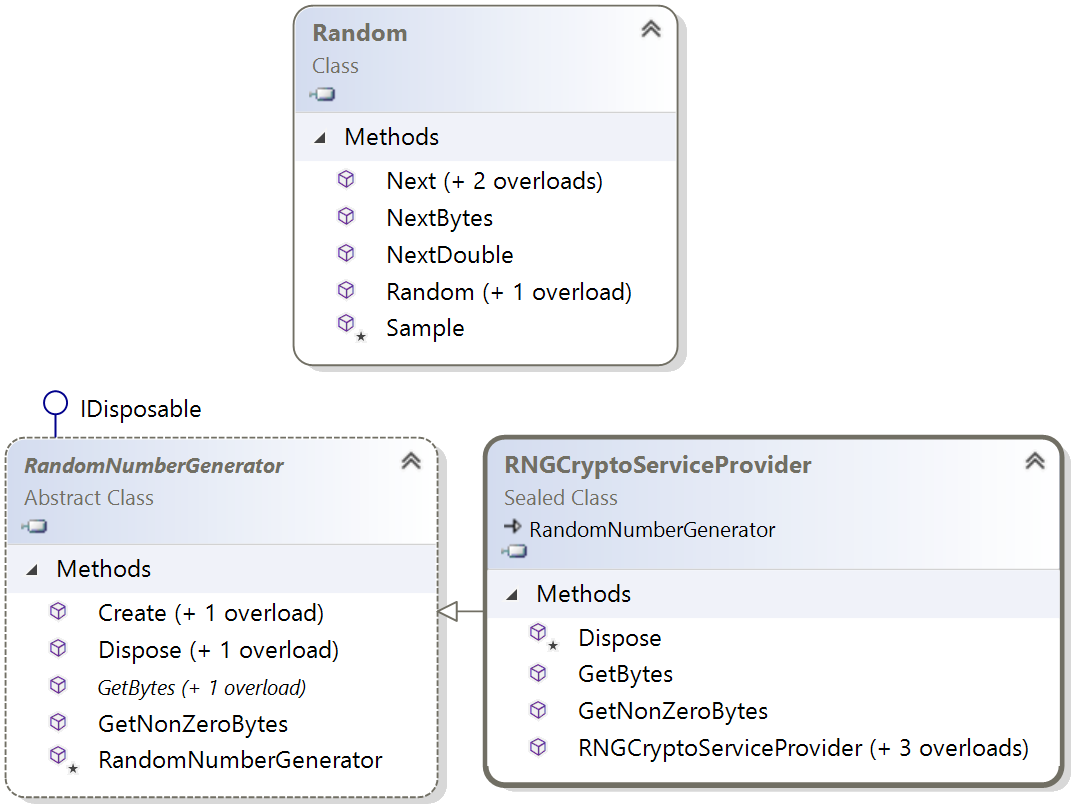
Generating random numbers for games
In scenarios that don't need truly random numbers, you can use the Random
class, as shown in the following code example:
var r = new Random();
Random
has a constructor with a parameter for specifying a seed value used to initialize a pseudo-random number generator, as shown in the following code:
var r = new Random(Seed: 12345);
Note
Good Practice
As you learned in Chapter 2, Speaking C#, parameter names should use camel case. The developer who defined the constructor for the Random
class broke this convention! The parameter name should be seed
, not Seed
.
Note
Shared seed values act as a secret key, so if you use the same random number generation algorithm with the same seed...