Practicing and exploring
Test your knowledge and understanding by answering some questions, getting some hands-on practice, and exploring the topics covered in this chapter with deeper research.
Exercise 2.1 – Test your knowledge
To get the best answer to some of these questions, you will need to do your own research. I want you to “think outside the book,” so I have deliberately not provided all the answers in the book.
I want to encourage you to get into the good habit of looking for help elsewhere, following the principle of “teach a person to fish.”
- What statement can you type in a C# file to discover the compiler and language version?
- What are the two types of comments in C#?
- What is the difference between a verbatim string and an interpolated string?
- Why should you be careful when using
float
anddouble
values? - How can you determine how many bytes a type like
double
uses in memory? - When should you use the
var
keyword? - What is the newest syntax to create an instance of a class like
XmlDocument
? - Why should you be careful when using the
dynamic
type? - How do you right-align a format string?
- What character separates arguments for a console app?
Appendix, Answers to the Test Your Knowledge Questions, is available to download from a link in the README in the GitHub repository: https://github.com/markjprice/cs11dotnet7.
Exercise 2.2 – Test your knowledge of number types
What type would you choose for the following “numbers”?
- A person’s telephone number
- A person’s height
- A person’s age
- A person’s salary
- A book’s ISBN
- A book’s price
- A book’s shipping weight
- A country’s population
- The number of stars in the universe
- The number of employees in each of the small or medium businesses in the United Kingdom (up to about 50,000 employees per business)
Exercise 2.3 – Practice number sizes and ranges
In the Chapter02
solution/workspace, create a console app project named Ch02Ex03Numbers
that outputs the number of bytes in memory that each of the following number types uses and the minimum and maximum values they can have: sbyte
, byte
, short
, ushort
, int
, uint
, long
, ulong
, float
, double
, and decimal
.
The result of running your console app should look something like Figure 2.10:
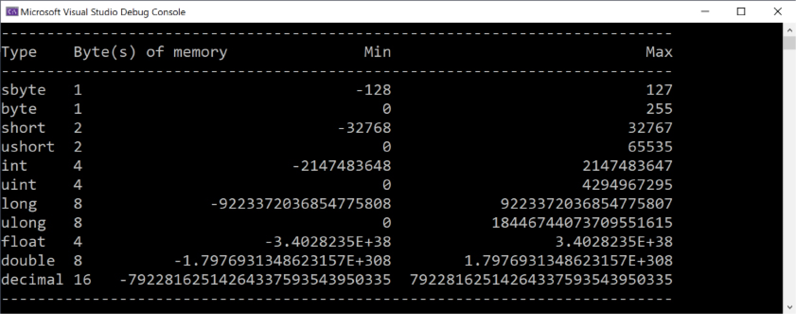
Figure 2.10: The result of outputting number type sizes
As a bonus exercise, output a row for the System.Half
type that was introduced in .NET 5.
Code solutions for all exercises are available to download or clone from the GitHub repository at the following link: https://github.com/markjprice/cs11dotnet7.
Exercise 2.4 – Explore topics
Use the links on the following page to learn more details about the topics covered in this chapter:
https://github.com/markjprice/cs11dotnet7/blob/main/book-links.md#chapter-2---speaking-c