Building an intelligent robot controller
Let's see how to build a robot controller using a genetic algorithm. We are given a map with the targets sprinkled all over it. The map looks like this:
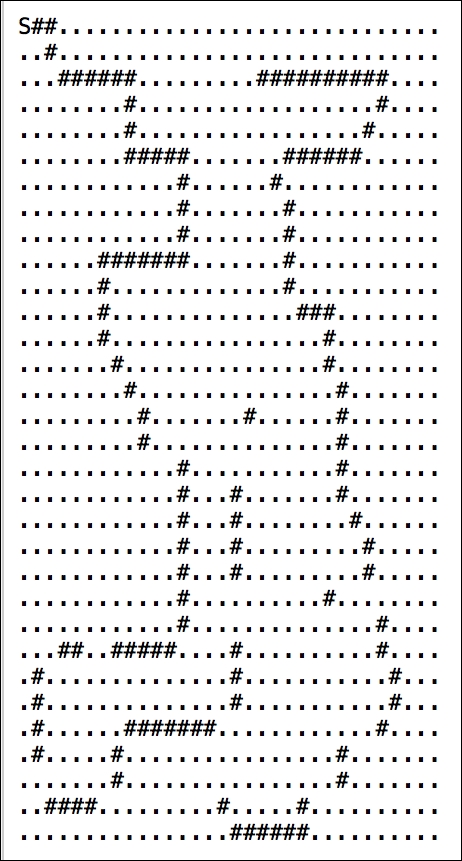
There are 124 targets in the preceding map. The goal of the robot controller is to automatically traverse the map and consume all those targets. This program is a variant of the artificial ant program given in the deap
library.
Create a new Python file and import the following:
import copy import random from functools import partial import numpy as np from deap import algorithms, base, creator, tools, gp
Create the class to control the robot:
class RobotController(object): def __init__(self, max_moves): self.max_moves = max_moves self.moves = 0 self.consumed = 0 self.routine = None
Define the directions and movements:
self.direction = ["north", "east", "south", "west"] self.direction_row =...