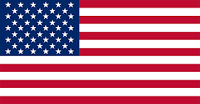

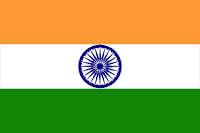
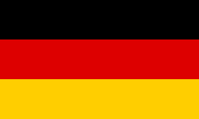
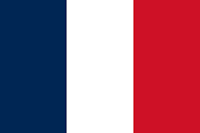

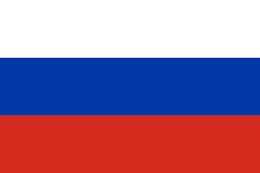
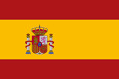



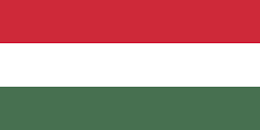
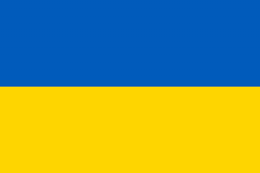
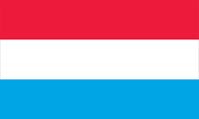

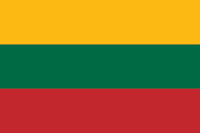

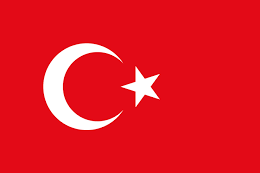


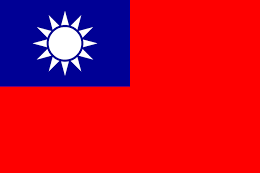
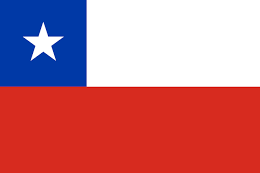

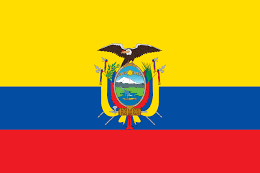
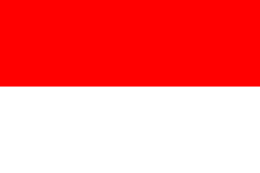
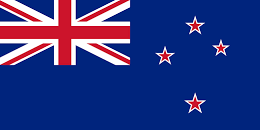
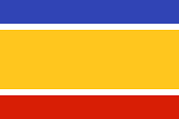
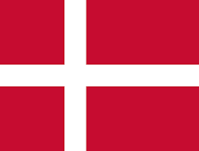
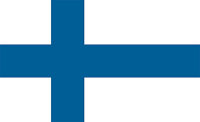


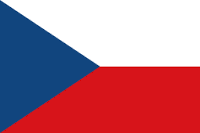
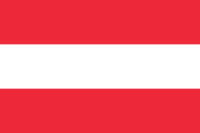
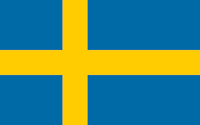
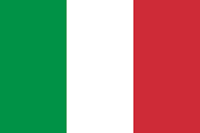
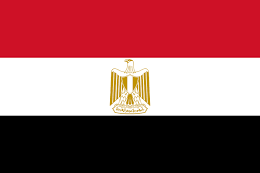

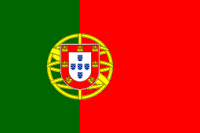
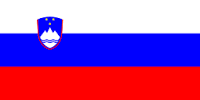

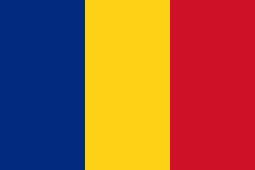
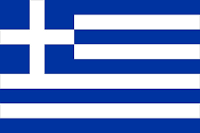


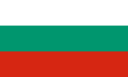
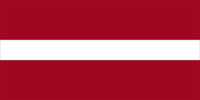
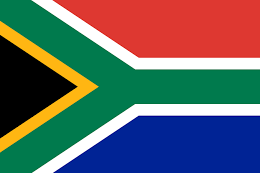
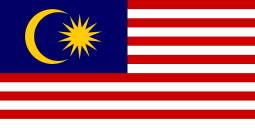



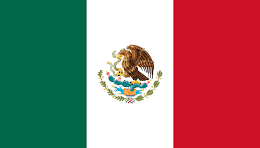
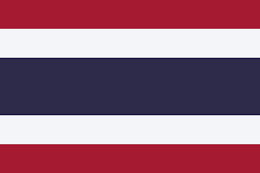
In the fast-paced world of game development, efficiency is key. Developers often find themselves repeating mundane tasks that can consume valuable time and lead to human error. Imagine if you could automate these repetitive tasks, freeing up your time to focus on more creative aspects of your game. In this article, we’ll explore how creating custom tools in Unity can transform your workflow, boost productivity, and reduce the risk of mistakes.
Custom tools are tailored solutions that address specific needs in your development process. Here’s why they are crucial:
Overview: We’ll walk through the process of creating a simple Unity editor tool to automate tasks like aligning game objects or batch renaming assets.
Custom tools address several common development challenges:
We've all encountered broken game objects in our scenes, and manually searching through every object to find missing script references can be tedious and time-consuming. One of the key advantages of editor scripts is the ability to create a tool that automatically scans all game objects and pinpoints exactly where the issues are.
. To use this script, simply place it in the Editor folder within your Assets directory. The script needs to be in the Editor directory to function properly. Here's the code that creates a new menu item, which you'll find in the Editor menu bar. What this script does is invoke the CheckDependencies method, which scans all game objects in the scene, checks for any missing components, and collects them in a list. The results are then displayed through the editor window using the OnGUI function.
public class ScriptDependencyChecker : EditorWindow
{
private static Vector2 scrollPosition;
private static string[] missingScripts = new string[0];
[MenuItem("Tools/Script Dependency Checker")]
public static void ShowWindow()
{
GetWindow<ScriptDependencyChecker>("Script Dependency Checker");
}
private void OnGUI()
{
if (GUILayout.Button("Check Script Dependencies"))
{
CheckDependencies();
}
if (missingScripts.Length > 0)
{
EditorGUILayout.LabelField("Objects with Missing Scripts:", EditorStyles.boldLabel);
scrollPosition = EditorGUILayout.BeginScrollView(scrollPosition, GUILayout.Height(300));
foreach (var entry in missingScripts)
{
EditorGUILayout.LabelField(entry);
}
EditorGUILayout.EndScrollView();
}
else
{
EditorGUILayout.LabelField("No missing scripts found.");
}
}
private void CheckDependencies()
{
var missingList = new System.Collections.Generic.List<string>();
GameObject[] allObjects = GameObject.FindObjectsOfType<GameObject>();
foreach (var obj in allObjects)
{
var components = obj.GetComponents<Component>();
foreach (var component in components)
{
if (component == null)
{
missingList.Add($"Missing script on GameObject: {obj.name}");
}
}
}
missingScripts = missingList.ToArray();
}
}
Now, let's head over to the Unity Editor and start using this tool. As shown in Image 01, you'll find the Script Dependency Checker under Tools | Script Dependency Checker in the menu bar.
Image 01 - Unity’s menu bar
When you click on it, a window will open with a button and a debug section that will display any game objects with missing script references, if found. You can see this in Image 02.
Image 02 - Script dependency window
After pressing the button, we discovered a game object named AudioManager with a missing script, as shown in Image 03.
Image 03 - Results of the Checker
Next, we can search for AudioManager in the hierarchy and address the issue by either reassigning the missing script or removing it entirely if it's no longer needed, as shown in Image 04.
Image 04 - Game Object with missing script
Creating custom tools in Unity not only enhances your productivity but also ensures a smoother and more efficient development process. As you experiment with building and implementing your tools, consider other repetitive tasks that could benefit from automation. Whether it’s organizing project folders or generating procedural content, the possibilities are endless.
By leveraging custom tools, you’ll gain more control over your development environment and focus on what truly matters—bringing your game to life. For more insights into Unity development and custom tools, check out my book, Mastering Unity Game Development with C#: Harness the Full Potential of Unity 2022 Game Development Using C#, where you’ll find in-depth guides and practical examples to further enhance your game development skills.
Mohamed Essam is a highly skilled Unity developer with expertise in creating captivating gameplay experiences across various platforms. With a solid background in game development spanning over four years, he has successfully designed and implemented engaging gameplay mechanics for mobile devices and other platforms. His current focus lies in the development of a highly popular multiplayer game, boasting an impressive 20 million downloads. Equipped with a deep understanding of cutting-edge technologies and a knack for creative problem solving, Mohamed Essam consistently delivers exceptional results in his projects.