DI is a software development technique where we can create objects that depend on other objects. DI helps the interaction between classes, but at the same time keeps the classes independent.
There are three types of classes in DI:
- A Service is a class that can be used (dependency).
- The Client is a class that uses dependency.
- The Injector passes the dependency (Service) to the dependent class (Client).
The three types of classes in DI are shown in the following diagram:
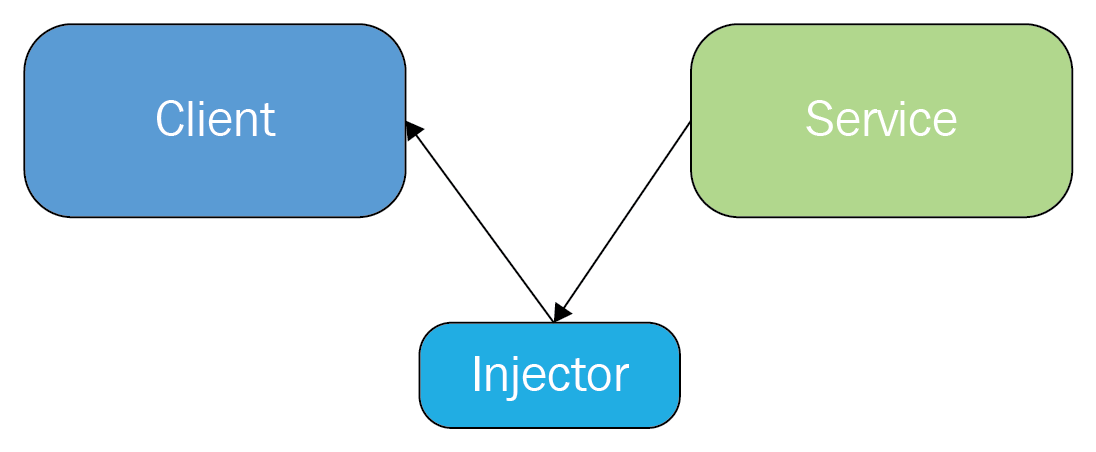
DI makes classes loosely coupled. This means that the creation of client dependencies is separated from the client's behavior, which makes unit testing easier.
Let's take a look at a simplified example of DI using Java code. In the following code, we don't have DI, because the client Car class is creating an object of the service class:
public class Car {
private Owner owner;
...