Adding jQuery programmatically to a web form
In addition to adding jQuery to web forms using the script block and the ScriptManager
control, the code-behind file can also emit the required script code. This recipe will demonstrate how this can be done.
Getting ready
- Create an ASP.NET Web Application project by navigating to File | New | Project | ASP.NET Web Application. Select the Empty template. Name the project
WebApplicationWithPageLoad
(or any other suitable name). - Add a new Web Form to the project and name it
Default.aspx
. - Add the jQuery library files to the Scripts folder.
- From the Solution Explorer tab, navigate to Default.aspx.vb (VB) or Default.aspx.cs (C#), which is the code-behind file for the web form. Open this file.
How to do it…
In the Page_Load
event handler of Default.aspx.vb
, use the RegisterClientScriptInclude
method to generate a script block on the page, as follows:
For VB, the code is as follows:
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load Page.ClientScript.RegisterClientScriptInclude("jquery", Page.ResolveUrl("~/Scripts/jquery-2.1.4.js")) End Sub
For C#, the code is as follows:
protected void Page_Load(object sender, EventArgs e) { Page.ClientScript.RegisterClientScriptInclude("jquery", Page.ResolveUrl("~/Scripts/jquery-2.1.4.js")); }
How it works…
The RegisterClientScriptInclude
method requires two parameters: the key and URL. It adds the script block with the path to the jQuery library in the <form>
element, as shown in the following screenshot. The Page.ResolveUrl
method is used to return a URL relative to the site root:
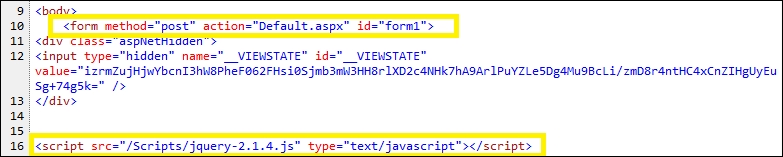
Since the jQuery library is added to the <form>
element, all the jQuery code should be written in the <form>
element instead of the <head>
element, preferably toward the end of the page before closing the <form>
element.
See also
The Adding jQuery to an empty ASP.NET web project using a script block recipe