Creating a read/process/write step
A read/process/write step is a common type of step where some data is read somewhere, processed in some way, and finally, saved somewhere else. In this recipe, we'll read a CSV file of users, increment their age, and save the modified users in a database as shown in the following image:
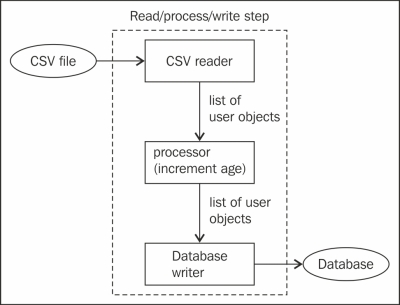
Getting ready
This is our CSV file of users, input_data.txt
:
Merlin, 333 Arthur, 37 Lancelot, 35 Tristan, 20 Iseult, 22 Mark, 56
For each line of the CSV file, we'll create a User
object. So, make sure that the User
class exists:
public class User { private String firstName; private int age; … }
Each User
object will be saved in the database. Make sure that the user
table exists:
CREATE TABLE user ( id BIGINT NOT NULL PRIMARY KEY AUTO_INCREMENT, first_name TEXT, age INT );
How to do it…
Follow these steps to process the CSV file:
In the Spring Batch configuration class, add a method returning a
LineMapper
object, which generates anUser
object from a line in the CSV file...