Slicing in NumPy is similar to Python lists. Indexing prefers to select a single value while slicing is used to select multiple values from an array.
NumPy arrays also support negative indexing and slicing. Here, the negative sign indicates the opposite direction and indexing starts from the right-hand side with a starting value of -1:
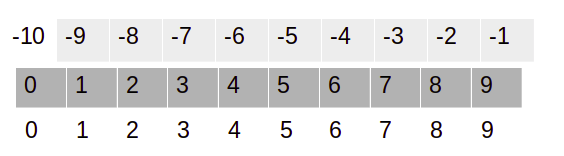
Let's check this out using the following code:
# Create NumPy Array
arr = np.arange(0,10)
print(arr)
Output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
In the slice operation, we use the colon symbol to select the collection of values. Slicing takes three values: start, stop, and step:
print(arr[3:6])
Output: [3, 4, 5]
This can be represented as follows:
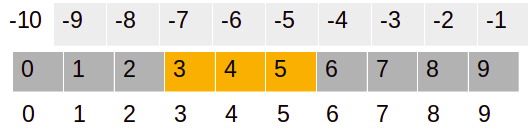
In the preceding example, we have used 3 as the starting index and 6 as the stopping index:
print(arr[3:])
Output: array([3, 4, 5, 6, 7, 8, 9])
In the preceding example, only the starting index is given. 3 is the starting index. This slice operation will select the values from the starting index...