Accessing a webcam with OpenCV
The first thing we'll do is use the webcam with OpenCV. This will be a little different from using the webcam with the Processing video library.
How to do it...
You need to start by importing the OpenCV library. Go to Sketch | Import Library | OpenCV. You'll see that the following line will be imported at the top of your sketch:
import hypermedia.video.*;
The next thing you need to do is to declare an OpenCV
object. In the setup()
function, we'll create the object and set up the camera with the capture()
method.
OpenCV opencv; void setup() { size( 640, 480 ); opencv = new OpenCV( this ); opencv.capture( width, height ); }
In the draw()
function, we'll read the image from the camera, flip it, and display it using the image()
function.
void draw() { opencv.read(); opencv.flip( OpenCV.FLIP_HORIZONTAL ); image( opencv.image(), 0, 0 ); }
The result of the sketch will look like the following screenshot:
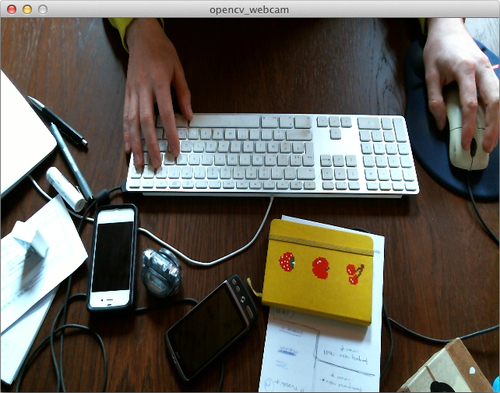
How it works...
You'll start by importing the OpenCV...