So far, we've seen how JavaScript can output information to the user. Consider the following code:
const Officer = function(name, rank, posting) {
this.name = name
this.rank = rank
this.posting = posting
this.sayHello = () => {
console.log(this.name)
}
}
const Riker = new Officer("Will Riker", "Commander", "U.S.S. Enterprise")
Now, if we execute Riker.sayHello(), we will see the following in the console:
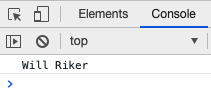
Figure 5.1 – Console output
Take a look for yourself in the chapter-5 directory in the repository: https://github.com/PacktPublishing/Hands-on-JavaScript-for-Python-Developers/blob/master/chapter-5/alerts-and-prompts/console.html.
OK, great. We have some console output, but that's not a very efficient way to get output, as users don't typically have the console open. There is a convenient method for output that, while not practical for a fully fledged web application, is useful for testing...