Chapter 5: Functional Programming
Activity 5 – Recursive Immutability
You are building an application in JavaScript and your team has been told that it cannot use any third-party libraries for security reasons. You must now use Functional Programming (FP) principles for this application and you need an algorithm to create immutable objects and arrays. Create a recursive function that enforces the immutability of objects and arrays at all levels of nesting with Object.freeze()
. For simplicity, you can assume that there are no null or classes nested in the objects. Write your function in activities/activity5/activity-test.js
. This file contains code to test your implementation.
To demonstrate forcing immutability in objects, follow these steps:
Open the activity test file at
activities/activity5/activity-test.js
.Create a function called
immutable
that takes in a single argument,data
.Check to see if
data
is not of typeobject
. If it is not, then return.Freeze the
data
object. You don't need to freeze non-objects.Loop through the object values with
object.values
and aforEach()
loop. Recursively call the immutable function for each.Run the code contained in the test file. If any tests fail, fix the bugs and rerun the test
Code:
activity-solution.js
function immutable( data ) { if ( typeof data !== 'object' ) { return; } Object.freeze( data ); Object.values( data ).forEach( immutable ); }
Snippet 5.11: Recursive immutability
Take a look at the following output screenshot below:
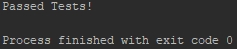
Figure 5.7 : Passed Tests output display
Outcome:
You have successfully demonstrated forcing immutability in objects.