Let’s define systems programming
Before we go into the nitty gritty details of systems programming, we need to set the stage. We need to have a common understanding of a couple of things. For instance, what does the term “systems programming” even mean? What is it for? Who is it for?
Let me get started with a definition.
Systems programming is the programming of systems. That might technically be correct, but I don’t think it helps us move forward.
Let us break it down: what is a system?
That one is easy. We have been building systems for ages, so we understand what we mean by a system.
Let me show you one definition:
A system is a set or arrangement of things that are related or connected so as to form a unity or organic whole. It is a collection of components or parts that interact with each other to function. This term is used in various fields such as physics, biology, computer science, and business management, each with slightly different connotations.
Great. But this definition is a bit broad. We might want to focus on computer science or software development. No problem; there are several definitions to choose from as well:
A system is a collection of software components that interact to perform a specific function or set of functions.
That is a lot better. If we dive into this a bit further, we can distinguish between different groups of systems:
- Software systems: This is an integrated set of software components that work together to carry out a specific function or set of functions. Those components can be a database server, micro-services, and a frontend. Those components form the complete system, such as a CRM system, source control repository system, and others like that.
- Operating systems (OSs): You probably know what an OS is. I think you have seen that term so often that there is a fair chance you didn’t even realize it is a system. But it most definitely is an OS that contains many parts and components, such as drivers, tools, helpers, and logs. Together, they deliver a system you as a user can use to run your software on, independently of the hardware.
- Distributed systems: We often refer to loosely connected components on a network as a distributed system. Each part is isolated from the others, but they must collaborate to achieve something worthwhile. For example, Azure DevOps runs on many different servers in the Azure cloud. All the components run potentially on different servers and machines, and these components can even be running in different parts of the world. However, they work together to form a complete solution for the end user.
- Embedded systems: An embedded system is usually a combination of hardware and software. The components are tightly coupled with each other. Developers usually write the software to match specific specifications so it uses the hardware best. Think, for instance, about the systems in your car. If you have a reasonably recent car, you undoubtedly have an entertainment system on board. The word “system” in “entertainment system” is a bit of a giveaway: it consists of many distinct components. There is very likely a device that can collect electromagnetic waves from the air (we call that a radio). That device is connected to some software that interprets those waves and turns them into an electrical signal to feed the speakers. Next to that, a component shows you, as the user, what you are listening to. I am sure you can find a lot of other systems in your car and probably in your TV, your phone, or your refrigerator.
There are many more examples, but I hope you see that a system always consists of individual components that are not useful on their own but, when combined, deliver a solution to a problem.
But hold on. We are not done yet.
Given these definitions and examples, you might think that the art of systems programming is just the programming of these systems, and you would not be wrong. But that is, in general, not what systems programming means. It most certainly is not what I mean by that.
Very, very roughly, we can divide software into two types:
- User-facing software: This is software written to be used by people. It has a user interface (UI) with buttons, lists, labels, and more. People interact with the software by using various means of input modalities.
- Software-facing software: This is software designed to be used by other software. There are no UIs since we have no users. We could say other components are the users, but when I say users, I mean people. Software-facing software interacts with other components through APIs, RPC (Remote Procedure Calls) calls, file transfer, and many other ways. No humans are involved in this.
It is the second type we are most interested in here in this book – software meant to be used by other software.
When is a system user-facing and when is it not?
It is not always clear when people are the primary users of some code or when other processes are. We could be very rigorous and say that anything with a UI is user-oriented; anything else is systems-oriented. That will make life easier for us if we want a clear definition. However, in the real world, the boundaries tend to blur.
Let me give you an example. Have a look at this Visual Studio Solution:
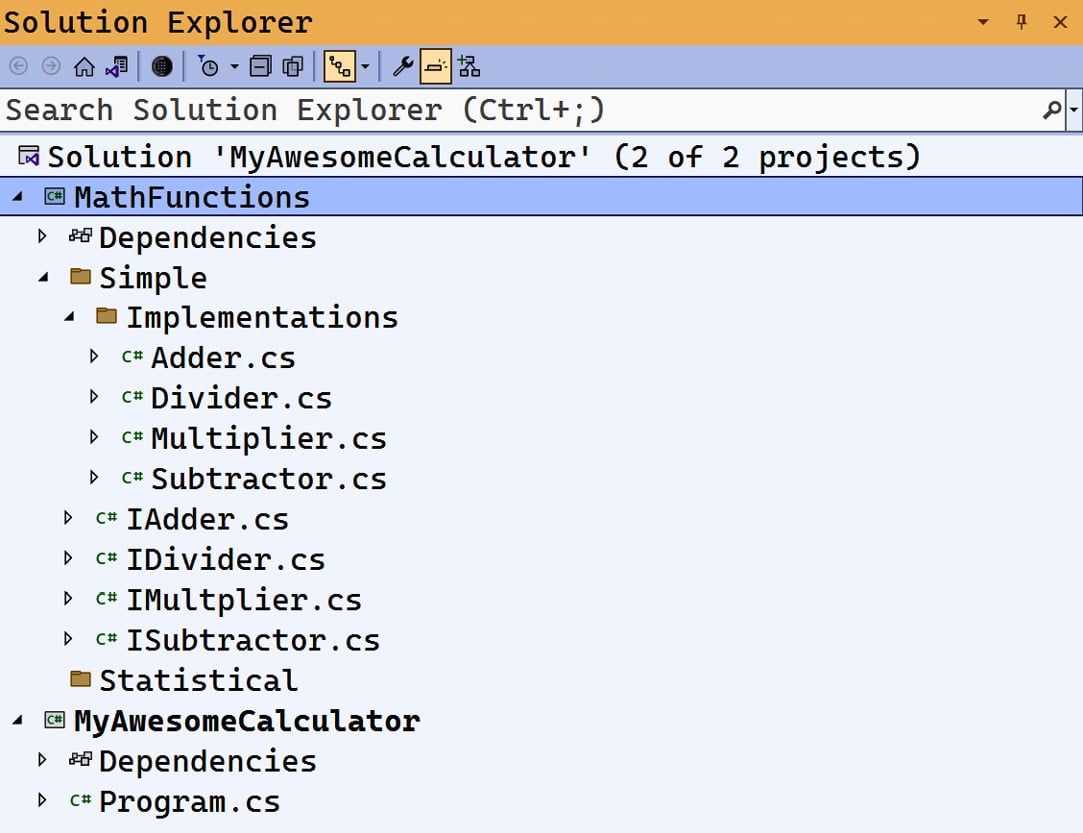
Figure 0.1: Solution Explorer with the Calculator project
We have a very, very simple solution here. It has a main program called MyAwesomeCalculator
that contains the main code. This is the entry point of our app, using the console as the UI. All logic is in the MathFunctions
class library. This is where the magic happens.
If we go back to our definition of Systems programming, we could say that writing the MathFunctions
class library is part of Systems programming. After all, no user will ever interact with the classes and interfaces in that library. It is the code in MyAwesomeCalculator
that actually uses it.
Great! This means writing the MathFunctions
library is systems programming! Well, not so fast. We might come to another conclusion if we look at the sequence diagram that explains the flow. Figure 0.2 shows this sequence diagram.
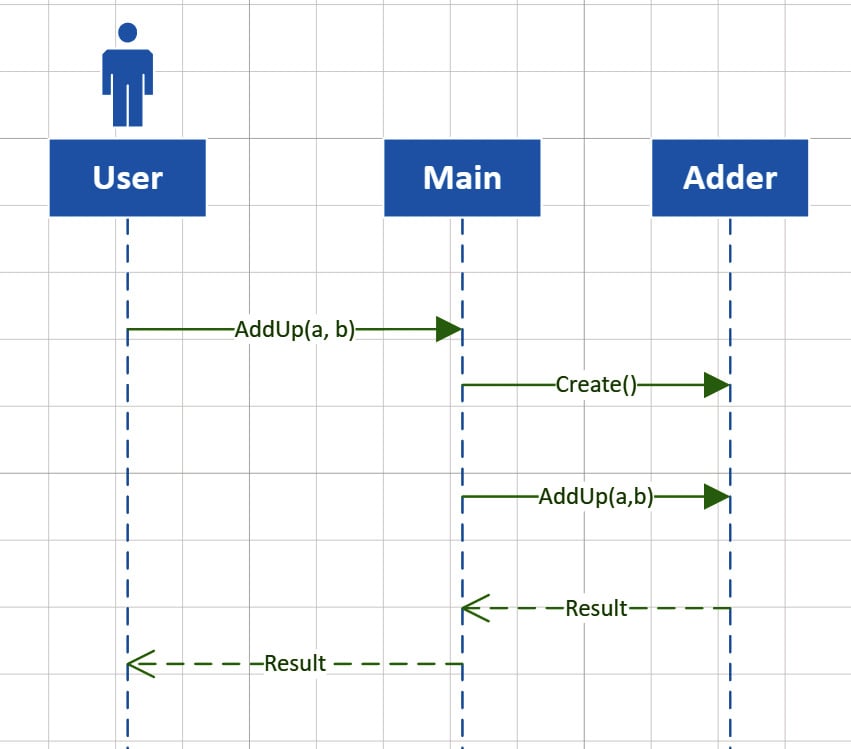
Figure 0.2: Sequence diagram for our calculations
As you can see in Figure 0.2, the user initiates an operation: they want to add up two numbers. They enter it in the UI of the Main
class. The Main
class then instantiates an instance of the Adder
class. After that creation, the Main
class calls the AddUp(a,b)
method. The result is passed back to the Main
class and shown to the user. After all this, we could discard the Adder
instance.
Great. Where are the boundaries? If we look at it this way, we could say that the code in Adder
and, thus, in the MathFunctions
library is immediately tied to user actions. So, it is user-facing code instead of systems-facing code.
I still like to use the question of who is using the code to determine what kind of software we are writing. But apparently, this is not enough. We need to go a bit deeper.
The code in MyAwesomeCalculator
and MathFunctions
are in separate assemblies. The user interacts with one assembly; the other is accessed through code only. But they can still be seen as one. If we run the application, the runtime creates AppDomain
for us.
AppDomain
in .NET is different than AppDomain
in .NET Framework. The latter had more ways to isolate code from each other. That was nice, but it was a typical Windows feature. That did not translate well to other platforms. So, to make .NET applications run on other platforms, they needed to redesign this. This results in AppDomain
being less restrictive than it used to be. Still, AppDomain
remains a logical boundary between different processes. Code runs in one app domain and cannot access other app domains directly.
Here, we have another clue: our MyAwesomeCalculator
app and the associated MathFunctions
assembly all run in the same AppDomain
. To the OS, they are one. Since we decided that actual people use the Main
method, the same applies to all other pieces of code in that particular AppDomain
.
Let’s rewrite our solution a bit. See the following screenshot.
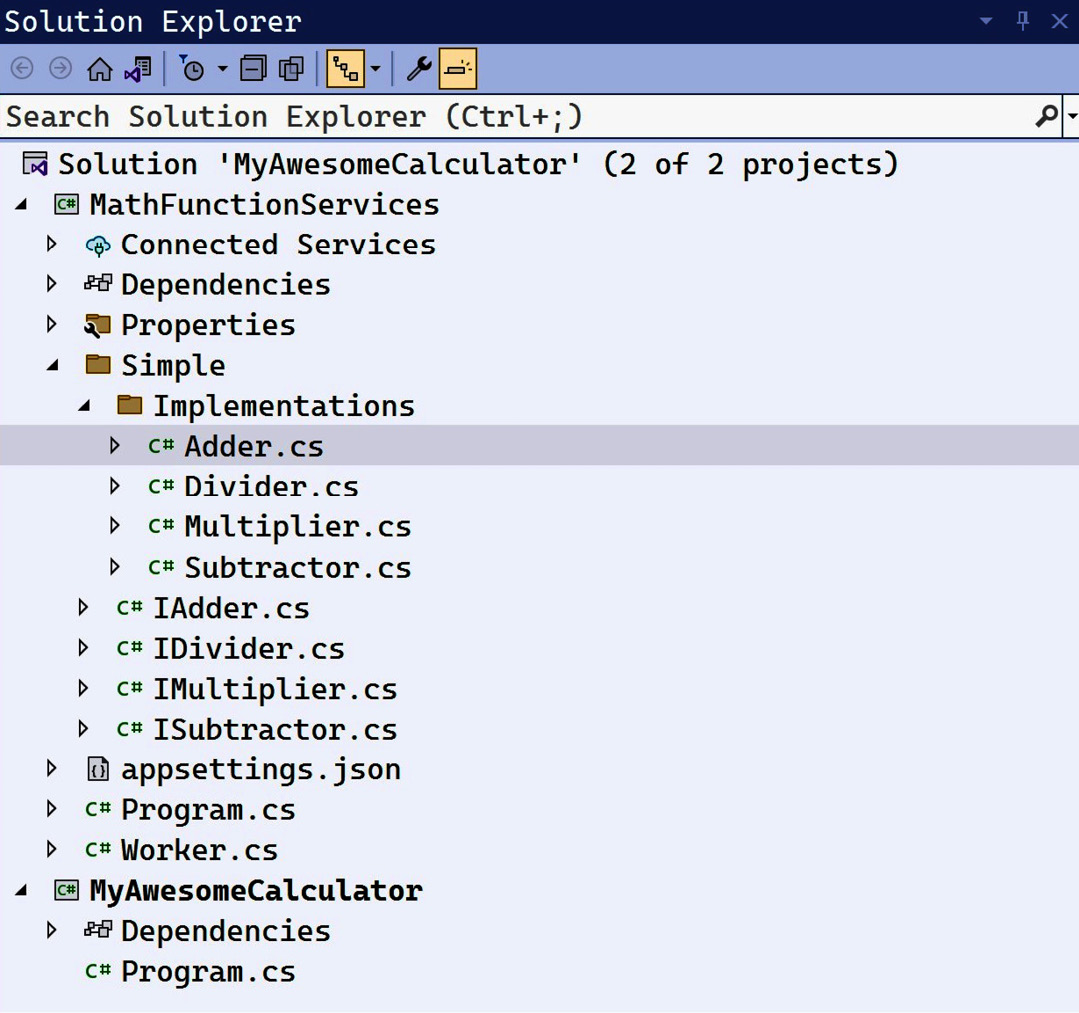
Figure 0.3: Our solution with a worker process
We removed the class library with the code that did all the work. Instead, we created a new project. That project is a worker process. Technically, I should have kept that class library and referenced that, but I wanted to keep things simple.
A worker process is a background process that runs all the time (not technically true, but for now, this is true enough). It just sits there doing nothing. Then, suddenly, something of interest happens, and it comes to life, does its job, and goes to idle mode again.
As shown in Figure 0.4, the sequence diagram in this case is also slightly different.
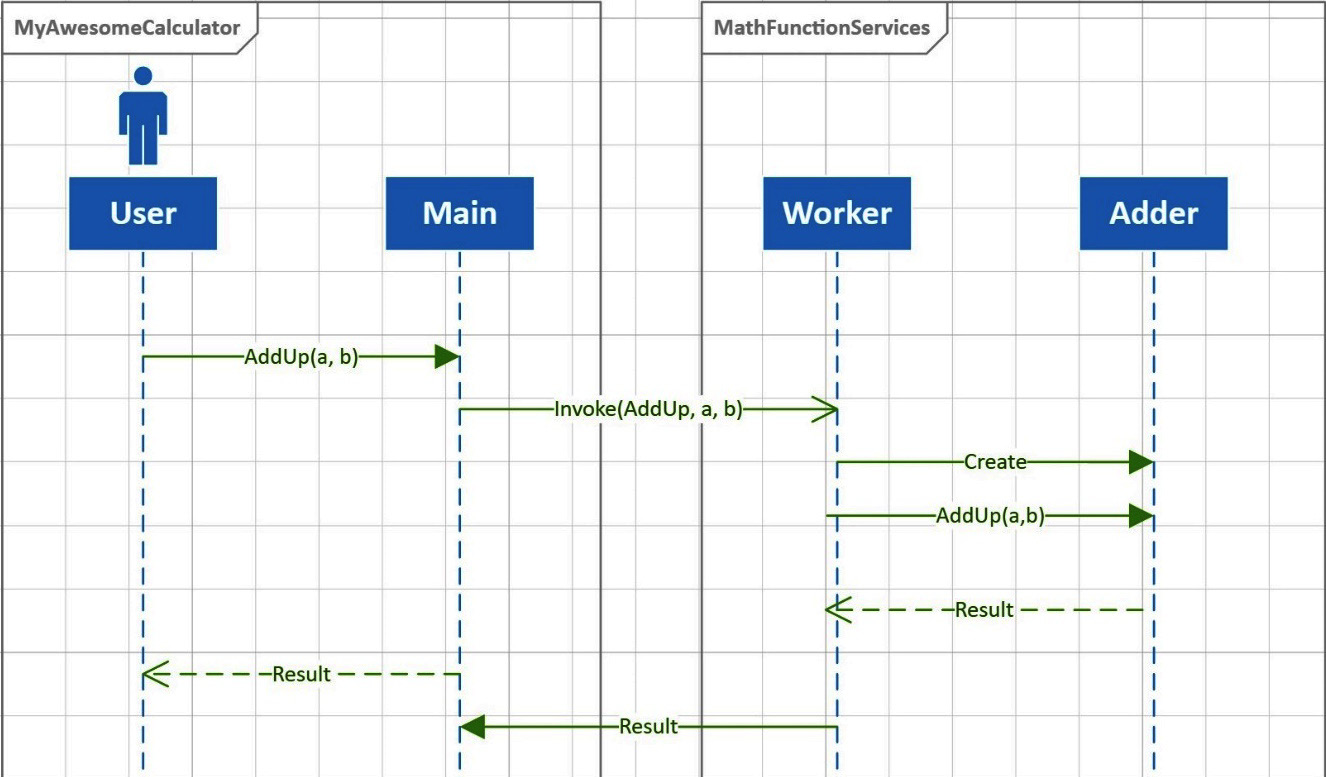
Figure 0.4: Sequence diagram for the new revised architecture
MyAwesomeCalculator
and the MathFunctionServices
worker are now independent of each other. They each run in their own AppDomain
. When the user wants to perform the calculation, they enter this in the UI, which invokes the service. The Worker
class picks up the command, creates an instance of the Adder
class, calls the AddUp
method, and then calls the MyAwesomeCalculator
again with the results.
As you can see, the calls between all classes are synchronous (designated by a line with a solid arrowhead) except for the call between Main
and Worker
. That is asynchronous (designated by a line and an open arrowhead).
That makes sense; the calculator cannot know whether the command has arrived or the service is listening. It just does a fire-and-forget, crosses its digital fingers, and hopes for the best.
This is more like it. This is genuinely writing software used by other software (I am talking about MathFunctionServices
here, not MyAwesomeCalculator
).
I have not shown you how the code in Main
calls Worker
and how the result flows back from Worker
to Main
. After all, they are in separate app domains. So, they cannot share memory, right? That is correct. I did not show you that. But do not worry. I have a couple of chapters dedicated to this.
It is important to realize that MathFunctionServices
does not have a UI in the ordinary sense of the word. No user ever touches this code. It lies there, dormant, until its services are required. If we compare that to the first example, we see the differences. That first example had all code loaded on the user’s demand, and it somehow all responded to the users’ actions.
A better definition
So, if we combine all of this, we can determine that systems programming is the art of writing components that can perform a function or a set of functions but interact only with other components.
That is what this book is all about. We will learn how to write software that is to be consumed by other software. That is a whole other way of looking at software, requirements, design considerations, and more compared to software meant for humans.
Writing software for software means other ways of thinking about communications, performance, memory usage, security, and so on. All those topics are covered here in this book. Now, you might say: “But wait a minute. Software written for users should also keep performance in mind!” You are right, but software communicating with software has unique needs.
Later chapters show how you can achieve the desired performance and explain why this is important. Let us agree that a component, potentially called thousands of times per second, could use more thought about performance than a screen with a button that a user might click once an hour. I am exaggerating here, but I am sure you get the point.
The same applies to memory consumption. I believe we should always write all software with memory consumption in mind. However, a component that gets used frequently by many other systems tends to be much more vulnerable to issues with memory than other software programs.
Performance and memory pressure are essential when we think about writing embedded systems. Embedded software usually runs on very limited hardware, so we have to try and take advantage of every trick in the book to get it running as fast as possible and using as little memory as possible.
As promised, we will spend much time looking at ways to communicate with these types of software.
To me, Systems programming is the purest form of software development. It is all about algorithms, tweaks, and trying out every trick in the book to get the most out of it. systems programming is the major league of software development. When you have this covered, all other software you write will also benefit from your newfound knowledge. What you learn when writing systems software will become second nature, and you will improve your overall software writing skills. Does this sound exciting? Then, let’s get started!