Generate DataContract from an XML Schema
In the contract-first development approach, one of the most important steps is to generate the data types used in the service from
XML Schemas, which represent the contract. As a unified distributed communication development platform, it is quite common to support such kind of DataContract
generation in WCF development.
Getting ready
If you are not yet familiar with the contract-first development approach, you can get a quick overview of it from Aaron Skonnard's MSDN article Contract-First Service Development at http://msdn.microsoft.com/en-us/magazine/cc163800.aspx.
How to do it...
Compose the XML schema that represents the
DataContract
types that will be used in our WCF service. The next screenshot shows a simple sample schema that contains a simple enum and a complex data type definition:Use WCF ServiceModel Metadata Utility Tool (Svcutil.exe) to generate
DataContract
type source code based on the XML Schema composed in step 1. Following is the sample command on how to use the Svcutil.exe tool:svcutil.exe /target:code /dataContractOnly /serializer:DataContractSerializer /importXmlTypesTestDataContractSchema.xsd
The generated
DataContract
is as follows:public enum LevelEnum : int { [System.Runtime.Serialization.EnumMemberAttribute()] Low = 2, ……………. } ………….. [System.Runtime.Serialization.DataContractAttribute(Name="TestData", Namespace="http://wcftest.org/datacontract")] public partial class TestData : object, System.Runtime.Serialization.IExtensibleDataObject { …………………. [System.Runtime.Serialization.DataMemberAttribute(IsRequired=true, Order=2)] public wcftest.org.datacontract.LevelEnum EnumProperty { } }
Use the generated
DataContract
in our WCF service as operation parameters or return type.[ServiceContract] public interface ITestService { [OperationContract] TestData GetData(); }
How it works...
The contract-first development approach is contract/schema driven; the developers need to author the metadata/contract of the service/data. For the previous example, TestDataContractSchema.xsd
provides the contract definition of two types that will be used in our WCF service.
Svcutil.exe is a very powerful tool provided in the .NET 3.5 SDK. If you're familiar with ASP .NET ASMX Web Service development, you will find it similar to the wsdl.exe tool. You can generate a WCF client proxy and export metadata from service code. Here we just use it to generate serialization code from the given XML Schema. In the previous sample, we specify DataContractSerializer
as the serialization type (you can also use
XMLSerializer
instead, if you prefer XML serialization-oriented code).
By capturing the service operation's underlying SOAP message on wire (refer to the next screenshot), we can find that the return value's XML payload conform to the XML Schema we provided as the generation source (TestDataContractSchema.xsd
):
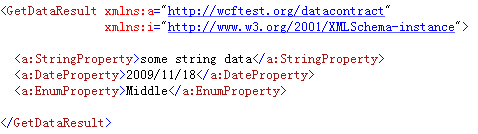
There's more...
The DataContract
we generated here includes two typical class types—a composite type and a simple enum type. In most scenarios, people will define much more complicated data types in their services, and WCF DataContractSerializer
does provide enough support for mapping between an XML Schema-based contract and .NET code-based types. You can get more information on type mapping in the MSDN document Data Contract Schema Reference, available at:
See also
Creating a typed service client in Chapter 4
Complete source code for this recipe can be found in the
\Chapter 1\recipe3\
folder