Using conditionals
In this section, we will level up our code and learn everything about conditionals. Conditionals allow us to perform different actions depending on the provided data. These different actions can be anything. We are allowed to program these actions ourselves. Here is an example script that prints something based on the player’s position during a race:
local playerPosition = 1
if playerPosition == 1 then
print("You are in the first place!")
end
The following text is printed in the Output frame when running this script: “You are in the first place!” However, when we change the value of the playerPosition
variable to any other number, nothing appears in Output. The reason nothing appears is because of our if
statement. To clear up any possible confusion, an if
statement is a conditional.
Everything you place between the if
and the then
part gives a Boolean. Everything between then
and end
is executed when this Boolean gives true
. If this is not the case, the code continues after the end
statement.
Two things were just said to explain the if
statement. We test to see whether this is correct in the following two sections.
Relational operators
We first said that everything between the if
and the then
statements has to return a true
Boolean. We can confirm this by making a print statement. Execute the following code:
local playerPosition = 1
print(playerPosition == 1)
Notice how the result of this script prints true
in Output? Now, if we change the value of our variable to two and execute the script, the result is false
. It is false
because we use the equal to (==
) operator, a relational operator. When using a relational operator, the outcome is always a Boolean.
Equal to (==
) is not the only relational operator that we have. The following is a list of all the relational operators that we have in Luau:
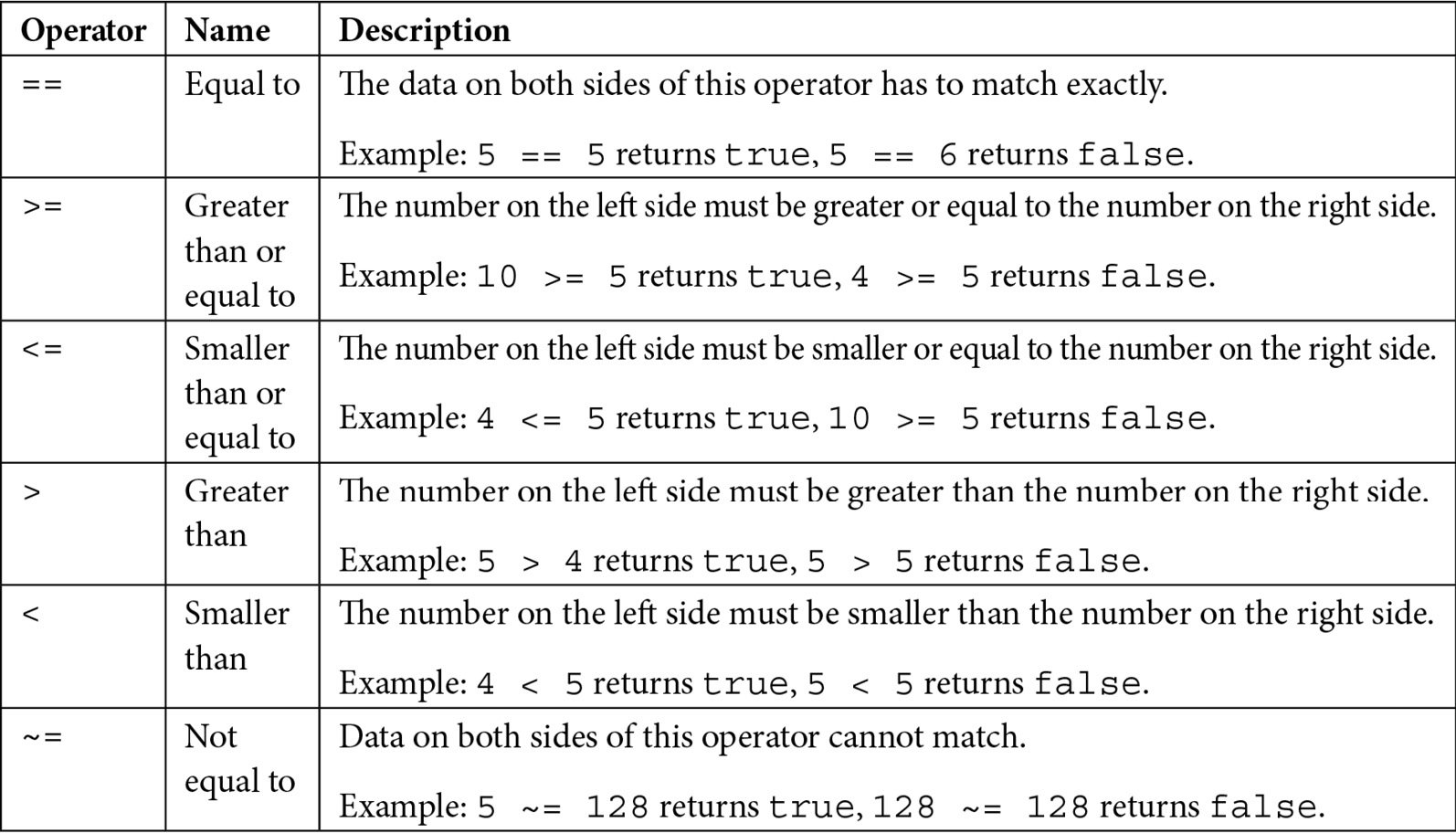
Table 1.1 – Relational operators explained
Now that we know how to make a simple if
statement, we can increase the complexity by adding an else
statement in our conditional. The next section explains how to do this.
if-else conditionals
The second thing that we said was that if the result of this relational operator comes back false
, everything between then
and end
is skipped, and the script continues behind end
. We can test this as well. We can put another print
behind end
and test whether it is executed, even when the result is false
. Execute the following code:
local playerPosition = 1
if playerPosition == 1 then
print("You are in the first place!")
end
print("Script completed.")
When we have our variable set to 1
, both prints appear in Output. However, if we change the value of our variable to 2
, only Script completed. ends up in the console.
Let us change our program. If a racer is in the top three, their position must be printed. If the racer is not in the top three, a motivational text is printed to support them. Let us try to implement this:
local playerPosition = 1
if playerPosition <= 3 then
print("Well done! You are in spot " .. playerPosition
.. "!")
end
print("You are not in the top three yet! Keep going!")
This code works, sort of. When we are in the fourth spot, the motivating text is printed. However, both messages are printed when we are in the top three. We can prevent this by making two if
statements below each other. So, your code would look like this:
local playerPosition = 1
if playerPosition <= 3 then
print("Well done! You are in spot ".. playerPosition ..
"!")
end
if playerPosition > 3 then
print("You are not in the top three yet! Keep going!")
end
This code works. It does what we want. However, there is a better way to do this. We also have an else
option. Remember when we said that the code continues after end
? There is an exception. If we add else
before end
, this part is executed first. When the code is false
, the else
section is always executed. Visualized, an if
-else
statement looks like this:
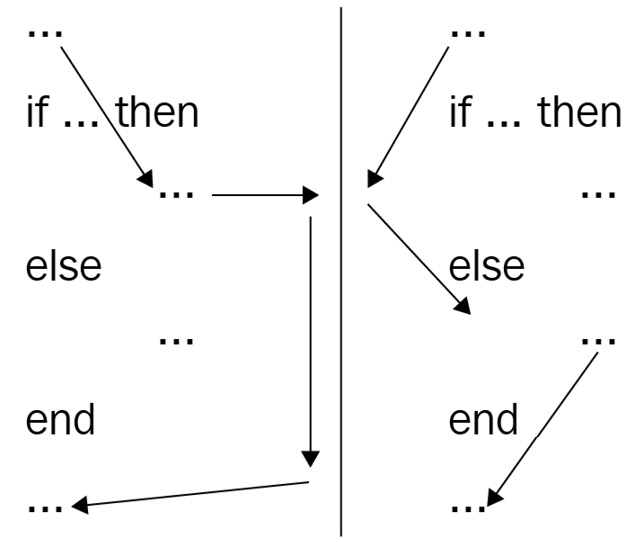
Figure 1.3 – An if-else statement visualized
Now that we know how an else
statement works, let us refactor our code to use this instead. Refactoring code is changing how it looks while keeping the same functionality. Our refactored code looks like this:
local playerPosition = 1
if playerPosition <= 3 then
print("Well done! You are in spot " .. playerPosition
.. "!")
else
print("You are not in the top three yet! Keep going!")
end
We have learned how to use the else
statement. In the next section, we will learn about another statement that we can use in conditionals. This other statement is elseif
.
Using elseif
This code looks a lot better. If we want another message for the players in spots four and five, this is possible. After all, they are almost there. We face another issue. We cannot add another if
statement in an already existing if
statement. Or can we? Let us take a look at this code:
local playerPosition = 1
if playerPosition <= 5 then
if playerPosition <= 3 then
print("Well done! You are in spot "..
playerPosition .. "!")
else
print("You are almost there!")
end
else
print("You are not in the top three yet! Keep going!")
end
This code does exactly what we want. However, it looks confusing. Our first if
statement checks whether the player’s position is below five. Then, it checks whether the position is below three. Right, we already had this. There are just two if
statements to get this now. Then, we get to the first else
statement. The number has to be either four or five to even get here. Then, we reach our final else
. This final else
is where all the numbers larger than five end up. As you can see, the system works. It just requires a bit more time to understand what is going on.
There is a better alternative. We already know about if
and else
. There is a combination of this, elseif
. An elseif
statement could be placed after if
and before else
. Because elseif
is also if
, we can add another expression.
Tip
The expression is the part between if
/ elseif
and then
.
Our code with elseif
would look like this:
local playerPosition = 1
if playerPosition <= 3 then
print("Well done! You are in spot " .. playerPosition
.. "!")
elseif playerPosition <= 5 then
print("You are almost there!")
else
print("You are not in the top three yet! Keep going!")
end
This looks much better! At a simple glance, you can see exactly what this code does.
Before we came up with the solution to use an elseif
statement, we tried using a nested if statement. In the following section, we take a deeper dive into these nested if
statements.
Nested if statements
There is still a slight issue with our code. What if you accidentally change the player’s position to zero? Or a negative number? As of right now, the system cannot handle this.
First, we must determine how many players there can be in a race to fix this problem. Let us say a race has a minimum of 1
player and a maximum of 8
players. This means that the player position has to be between those numbers. These numbers can be variables. As a matter of fact, they can even be constants. The minimum and the maximum number of players never change while the script runs.
Besides the new constants, there are multiple ways of implementing this feature. Here are two correct methods of implementing constants:
local MINIMUM_PLAYERS = 1
local MAXIMUM_PLAYERS = 8
local playerPosition = 1
-- Checking player's position
if playerPosition >= MINIMUM_PLAYERS and playerPosition <= 3 then
print("Well done! You are in spot " .. playerPosition
.. "!")
elseif playerPosition >= MINIMUM_PLAYERS and playerPosition <= 5 then
print("You are almost there!")
elseif playerPosition >= MINIMUM_PLAYERS and playerPosition <= MAXIMUM_PLAYERS then
print("You are not in the top three yet! Keep going!")
else
warn("Incorrect player position [" .. playerPosition ..
"]!")
end
Let us take a look at this code. The first thing that we notice is the text behind --
. This is something that has not been mentioned yet. By using --
, you can make comments in your code. These comments do not get executed, but they help other developers when reading your code.
The second new thing is the warn()
function used in the else
statement. The warn()
function is almost identical to the print()
function. The only difference is that the color in Output turns orange. This is because you use this method to warn, as the name implies. When you see a warning in your Output, usually someone did something that the system prevented, such as having a wrong playerPosition
in our case.
Something else that we have not seen before is the and
operator in an if
statement. When we learned that the true and true
expression gives true
, it probably did not feel like something substantial. However, we have the same thing in our if
statement right now. So, it is essential to understand.
Tip
If you have forgotten how the and
or the or
operator works, it might be wise to reread the Logical operators section in this chapter.
The only thing that could be improved is the expression, which checks whether the position is higher than the minimum multiple times. Therefore, in this scenario, it is not an ideal solution. We can change this by having an if
statement inside another one. Your code would look like this:
local MINIMUM_PLAYERS = 1
local MAXIMUM_PLAYERS = 8
local playerPosition = 1
-- Checking if the player's position is a valid number
if playerPosition >= MINIMUM_PLAYERS and playerPosition <= MAXIMUM_PLAYERS then
-- Getting correct message based on player's position
if playerPosition <= 3 then
print("Well done! You are in spot "..
playerPosition .. "!")
elseif playerPosition <= 5 then
print("You are almost there!")
else
print("You are not in the top three yet! Keep
going!")
end
else
-- The position of the player is not valid
warn("Incorrect player position [" .. playerPosition ..
"]!")
end
This example uses an if
statement within an if
statement. if
statements within if
statements are perfectly allowed. There is even a name for this. The if
statement within the other statement is called a nested if statement. It is good practice not to have more than three if
statements within each other. Later in the book, we will learn ways to reduce the amount of nested if
statements, such as by using functions and loops. You can find this information in Chapter 2, Writing Better Code.
We now know how to use conditionals in our code properly. We can use if
, elseif
, and else
statements. If you practiced yourself, you might have run into issues where certain variables were not accessible throughout your entire script. These variables were not accessible because of scopes. In the next section, we take a look at what these scopes are.