Example of label propagation
We can implement the algorithm in Python, using a test bidimensional dataset:
from sklearn.datasets import make_classification
nb_samples = 100
nb_unlabeled = 75
X, Y = make_classification(n_samples=nb_samples, n_features=2, n_informative=2, n_redundant=0, random_state=1000)
Y[Y==0] = -1
Y[nb_samples - nb_unlabeled:nb_samples] = 0
As in the other examples, we set y = 0 for all unlabeled samples (75 out of 100). The corresponding plot is shown in the following graph:
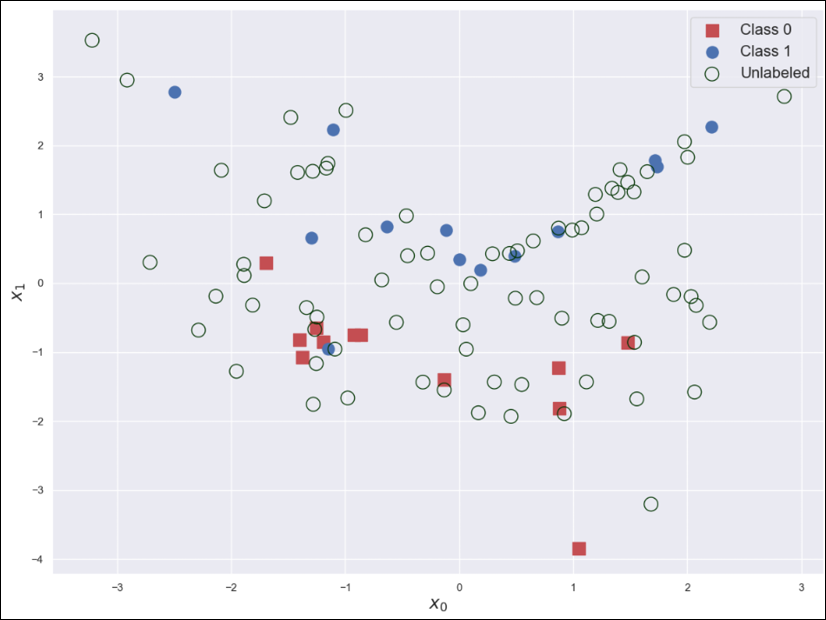
Partially labeled dataset
The dots marked with a cross are unlabeled. At this point, we can define the affinity matrix. In this case, we compute it using both methods:
from sklearn.neighbors import kneighbors_graph
nb_neighbors = 2
W_knn_sparse = kneighbors_graph(X, n_neighbors=nb_neighbors, mode='connectivity', include_self=True)
W_knn = W_knn_sparse.toarray()
The KNN matrix is obtained using the scikit-learn function kneighbors_graph...