The Object Class
Java provides a special class called Object, from which all classes implicitly inherit. You don't have to manually inherit from this class because the compiler does that for you. Object is the superclass of all classes:
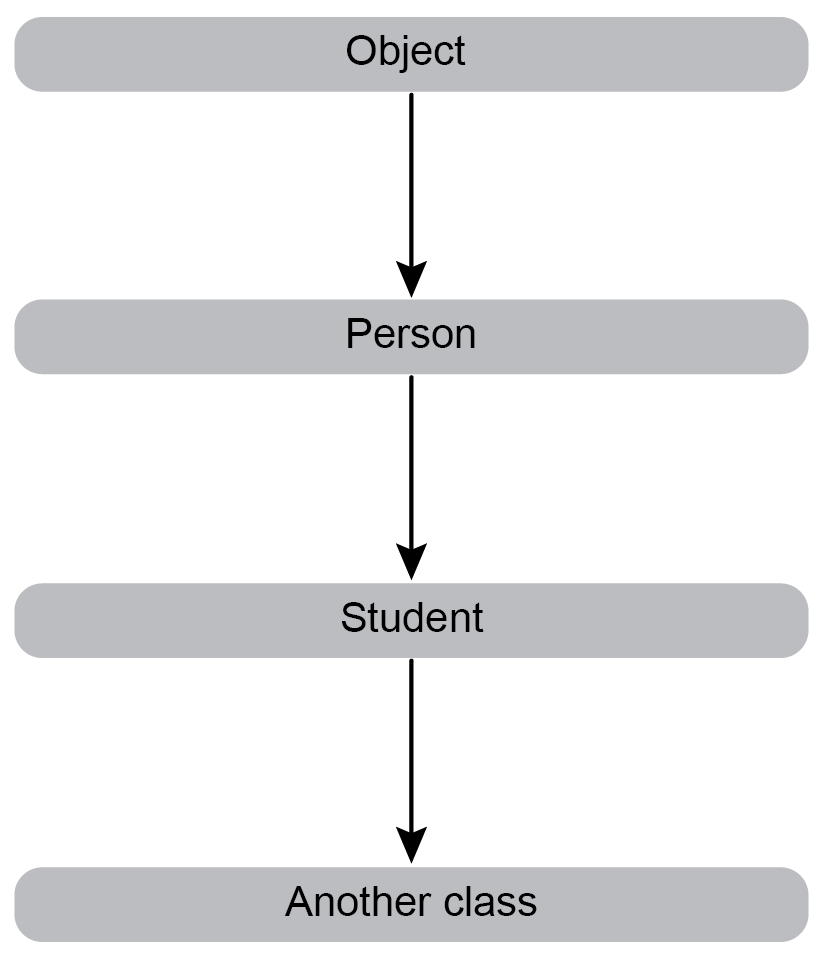
Figure 5.4: Superclass Object
This means that any class in Java can be upcast to Object:
Object object = (Object)person; Object object1 = (Object)student;
Likewise, you can downcast to the original class:
Person newPerson = (Person)object; Student newStudent = (Student)object1;
You can use this Object class when you want to pass around objects whose type you don't know. It's also used when the JVM wants to perform garbage collection.