Introducing the Django MVT architecture
There are various methodologies and approaches to design and code web applications. One approach involves consolidating all code into a single file to construct the entire web application. However, finding errors within such a file, often comprising thousands of lines of code, can be incredibly challenging. Alternatively, other strategies distribute code across different files and directories. Additionally, some approaches segment an application into multiple smaller applications dispersed across several servers, although managing the distribution of these servers presents its own set of challenges.
Organizing your code effectively presents challenges. This is why developers and computer scientists have created software architectural patterns. Software architectural patterns offer structural frameworks or layouts to address common software design issues. By leveraging these patterns, start-ups and inexperienced developers can avoid reinventing solutions for every new project. Various architectural patterns exist, including Model-View-Controller (MVC), Model-View-Template (MVT), layers, service-oriented, and microservices. Each pattern comes with its own set of pros and cons. Many frameworks, such as Django, adhere to specific patterns in constructing their applications.
In the case of Django, Django is based on the MVT pattern. This pattern is similar to MVC but with some differences in the responsibilities of each component:
- Models: The model represents the data structure. In Django, models are Python classes that define the structure of the data and how it interacts with the database. Models handle tasks such as querying a database, performing CRUD (Create, Read, Update, Delete) operations, and enforcing data validation. In the case of the Movies Store app, Movie, Review, Order and the other classes from our class diagram will be coded as Django models.
- Views: Views in Django are responsible for processing user requests and returning appropriate responses. Views typically receive HTTP requests from clients, fetch data from the database using models, and render templates to generate HTML responses. In Django, views are Python functions or classes that accept HTTP requests and return HTTP responses. In the case of the Movies Store app, we will create views and functions to handle the movies, accounts, and cart, among others.
- Templates: Templates are used to generate HTML dynamically. They contain the application’s user interface and define how data from the views should be displayed to the users. In the case of the Movies Store app, we will create a template to allow users to log in, a template to list movies, and a template to display the shopping cart, among others.
The MVT pattern offers several benefits such as enhanced code separation, facilitated collaboration among multiple team members, simplified error identification, increased code reusability, and improved maintainability. Figure 1.11 illustrates the software architecture of the Movies Store, which we will develop throughout this book. While it may seem overwhelming now, you will understand the intricacies of this architecture by the book’s conclusion. We will delve deeper into the architecture in the final chapters.
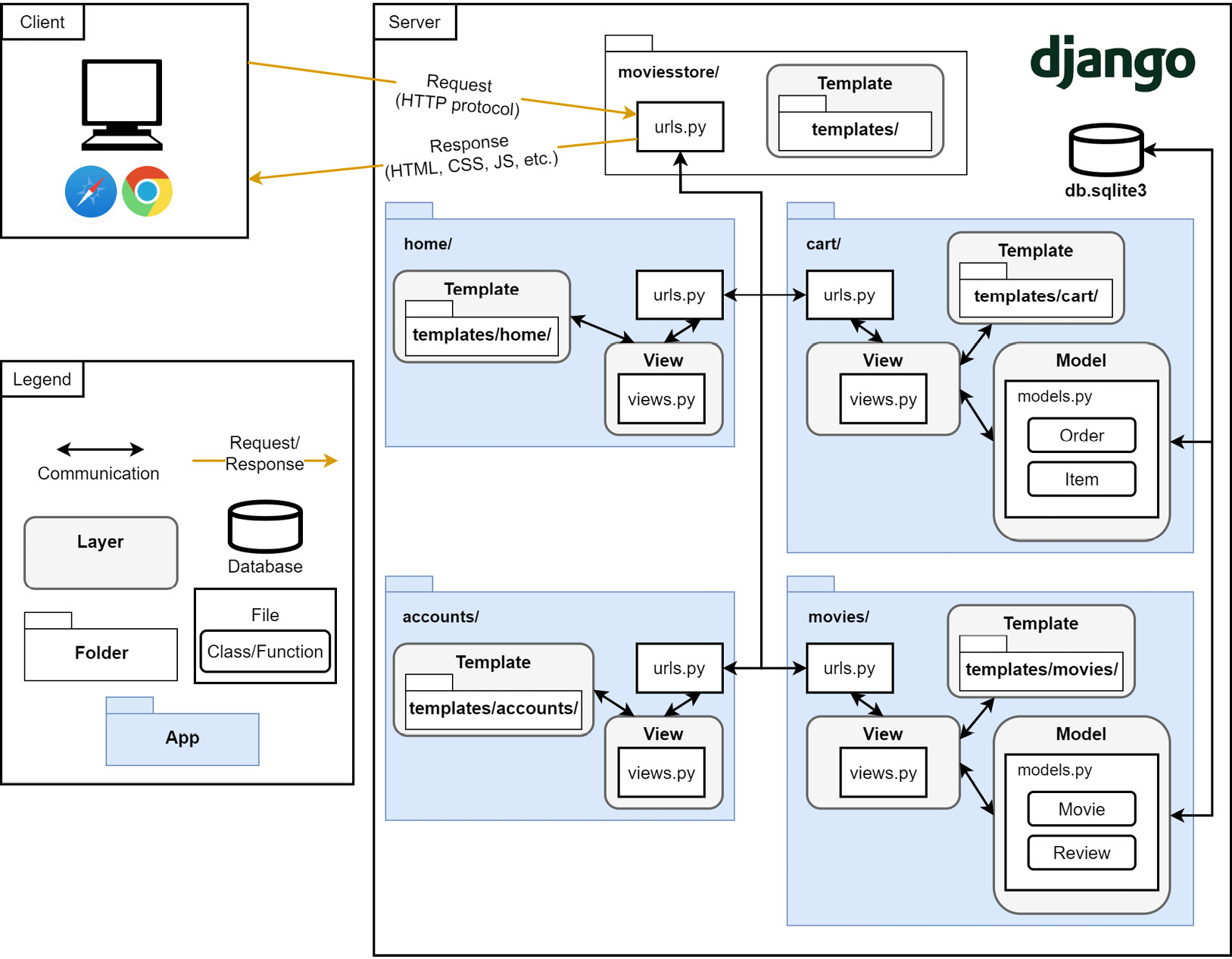
Figure 1.11 – The Movies Store software architecture diagram
Let’s briefly analyze this architecture:
- Positioned on the left are the clients, which are the users of our application, who use browsers on mobile or desktop devices. These clients establish connections with the application via the Hypertext Transfer Protocol (HTTP), providing users with a means to interact with our web application.
- On the right side, we have the server, which hosts our application code.
- All client interactions first pass for a project-level URL file called
urls.py
. This file is located in the main project folder calledmoviesstore/
. URLs will be explored in Chapter 2. This project folder also contains atemplates/
folder in which we will design a reusable base template. Base templates will be explored in Chapter 3. - The project-level URL file passes the interaction to an app-level URL file. For this project, we will design and implement four Django apps – home, movies, cart, and accounts. Django apps will be explored in Chapter 2.
- Each app-level URL file passes the interaction to a
views.py
file. Views will be explored in Chapter 2. - Views communicate with models, if required, and pass information to the templates, which are finally delivered to the clients as HTML, CSS, and JS code. Templates will be explored in Chapter 2, and models will be explored in Chapter 5.
In Figure 1.11, the Model, View, and Template layers are highlighted in gray, representing the common architectural pattern used in Django, which will be utilized throughout this book. We have four models corresponding to the classes defined in our class diagram (as previously shown in Figure 1.6). The user model does not appear in this diagram because we will reuse a built-in Django user model.
Therefore, as mentioned earlier, there are different approaches to implementing web applications with Django. There are even different ways to implement a Django MVT architecture. In the following chapters, we will see the advantages of adopting an MVT architecture, as presented in Figure 1.11.