Drawing a flag
Using the knowledge of Bezier curves you can develop various examples of your own. Here is a recipe whose output looks like this:
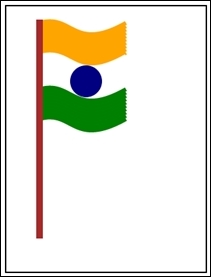
How to do it...
If you observe the drawing of a flag, you can easily understand that it's drawn using a combination of a line, a Bezier curve, and a circle. Here is our recipe:
<html> <head> <title>A Flag</title> <script> function init() { can = document.getElementById("MyCanvasArea"); ctx = can.getContext("2d"); var xstart = 50; var ystart = 25; var xctrl1 = 100; var yctrl1 = 10; var xctrl2 = 100; var yctrl2 = 70; var xend = 180; var yend = ystart; //10 curves having orange color for(i=1;i<=10;i++) { drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend, yend,"orange"); ystart+=5;yend+=5;yctrl1+=5;yctrl2+=5; } //10 curves having white color for(j=1;j<=10;j++) { drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend,yend,"white"); ystart+=5;yend+=5;yctrl1+=5;yctrl2+=5; } //10 curves having green color for(j=1;j<=10;j++) { drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend,yend,"green"); ystart+=5;yend+=5;yctrl1+=5;yctrl2+=5; } //the ashoka wheel we draw two arcs and lines x1=120; y1=113; drawArc(x1,y1,22,0,360,false,"navy"); //draw a stand for the flag drawLine(50,350,50,20,10,"brown"); } function drawBezierCurve(xstart,ystart,xctrl1,yctrl1,xctrl2,yctrl2,xend,yend,color,width) { ctx.strokeStyle=color; ctx.lineWidth=6; ctx.beginPath(); ctx.moveTo(xstart,ystart); ctx.bezierCurveTo(xctrl1,yctrl1,xctrl2,yctrl2,xend,yend); ctx.stroke(); } function drawArc(xPos,yPos,radius,startAngle,endAngle,anticlockwise,lineColor) { var startAngle = startAngle * (Math.PI/180); var endAngle = endAngle * (Math.PI/180); var radius = radius; ctx.strokeStyle = lineColor; ctx.fillStyle="navy"; ctx.lineWidth = 4; ctx.beginPath(); ctx.arc(xPos,yPos,radius,startAngle,endAngle,anticlockwise); ctx.fill(); ctx.stroke(); } function drawLine(xstart,ystart,xend,yend,width,color) { ctx.beginPath(); ctx.strokeStyle=color; ctx.lineWidth=width; ctx.moveTo(xstart,ystart); ctx.lineTo(xend,yend); ctx.stroke(); ctx.closePath(); } </script> </head> <body onload="init()"> <canvas id="MyCanvasArea" width ="300" height="400" style="border:2px solid black"> Your browser doesn't currently support HTML5 Canvas. </canvas> </body> </html>
How it works...
In the recipe, you will notice that the function to draw a Bezier curve is called 10 times through a loop for different coordinates and colors. Other than this, a vertical line with a thickness of 10
is drawn. For this, the function drawLine()
is called specifying the appropriate parameters.