A circular linked list can have only one reference direction (as with a linked list) or a double reference (as with a doubly linked list). The only difference between a circular linked list and a linked list is that the last element's next (tail.next) pointer does not make a reference to undefined, but to the first element (head), as we can see in the following diagram:
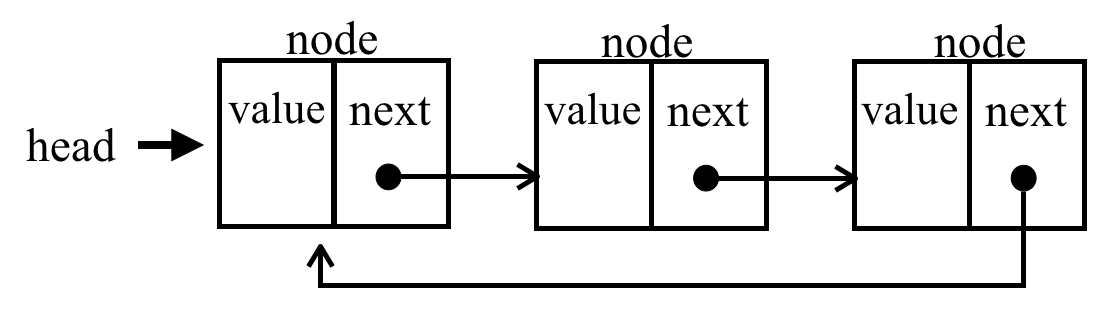
A doubly circular linked list has tail.next pointing to the head element, and head.prev pointing to the tail element:
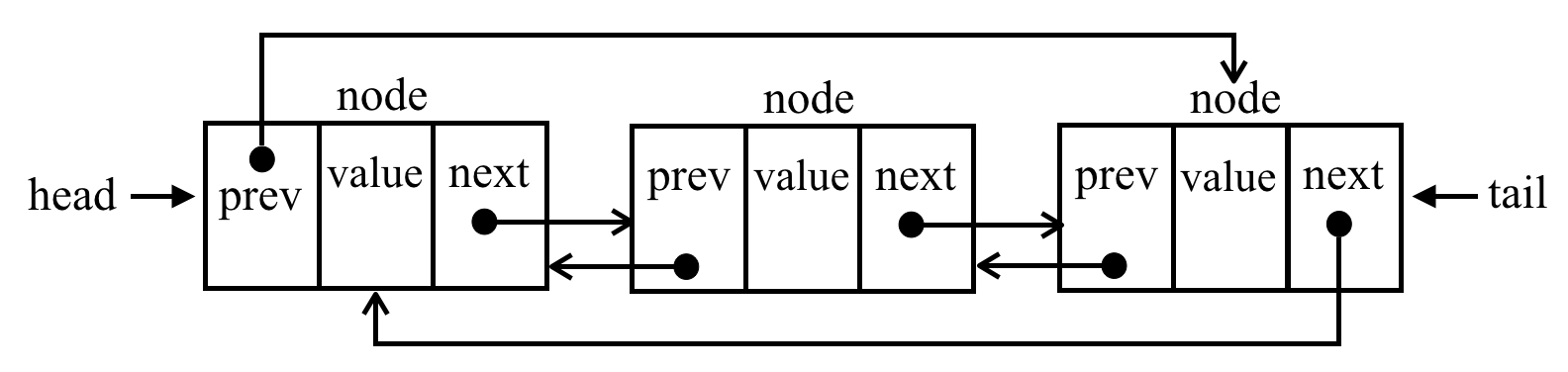
Let's check the code to create the CircularLinkedList class:
CircularLinkedList extends LinkedList {
constructor(equalsFn = defaultEquals) {
super(equalsFn);
}
}
The CircularLinkedList class does not need any additional properties, so we can simply extend the LinkedList class and overwrite the required methods to apply the special behavior.
We will overwrite...