If  is a matrix and
is a vector, you can solve the linear equation system
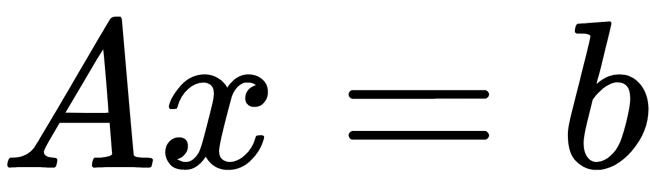
by using the function solve from the linear algebra submodule numpy.linalg:
from numpy.linalg import solve x = solve(A, b)
For example, to solve
the following Python statements are executed:
from numpy.linalg import solve A = array([[1., 2.], [3., 4.]]) b = array([1., 4.]) x = solve(A, b) allclose(dot(A, x), b) # True allclose(A @ x, b) # alternative formulation
The command allclose is used here to compare two vectors. If they are close enough to each other, this command returns True. Optionally a tolerance value can be set. For more methods related to linear equation systems, see Section 4.9: Linear algebra methods in SciPy.
Now, you have seen the first and essential way of how to use arrays in Python. In the following sections, we'll show you more details and the underlying principles.