Going back to the proposed file structure from earlier, your project should look like this:
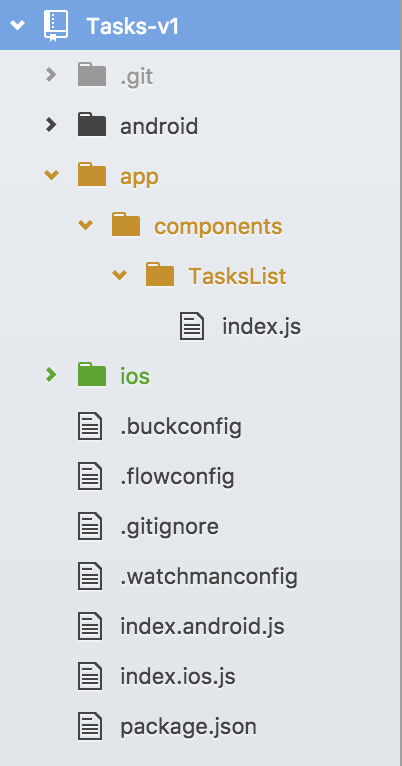
Let's start by writing our first component--the TasksList module.
The first thing we will need to do is import our dependency on React:
import React, { Component } from 'react';
Then, we'll import just the building blocks we need from the React Native (react-native) library:
import {
ListView,
Text
} from 'react-native';
Now, let's write the component. The syntax for creating a new component in ES6 is as follows:
export default class TasksList extends Component {
...
}
From here, let's give it a constructor function to fire during its creation:
export default class TasksList extends Component {
constructor (props) {
super (props);
const ds = new ListView.DataSource({
rowHasChanged: (r1, r2) => r1 !== r2
});
this.state = {
dataSource: ds.cloneWithRows([
'Buy milk',
'Walk the dog',
'Do laundry',
'Write the first chapter of my book'
])
};
}
}
Our constructor sets up a dataSource property in the TasksList state as equal to an array of hardcoded strings. Again, our first goal is to simply render a list on the screen.
Next up, we'll utilize the render method of the TasksList component to do just that:
render () {
return (
<ListView
dataSource={ this.state.dataSource }
renderRow={ (rowData) =>
<Text> { rowData } </Text> }
/>
);
}
Consolidated, the code should look like this:
// Tasks/app/components/TasksList/index.js
import React, { Component } from 'react';
import {
ListView,
Text
} from 'react-native';
export default class TasksList extends Component {
constructor (props) {
super (props);
const ds = new ListView.DataSource({
rowHasChanged: (r1, r2) => r1 !== r2
});
this.state = {
dataSource: ds.cloneWithRows([
'Buy milk',
'Walk the dog',
'Do laundry',
'Write the first chapter of my book'
])
};
}
render () {
return (
<ListView
dataSource={ this.state.dataSource }
renderRow={ (rowData) =>
<Text>{ rowData }</Text> }
/>
);
}
}
Great! That should do it. However, we need to link this component over to our application's entry point. Let's hop over to index.ios.js and make some changes.