Strings: Concatenation, Methods, and input()
You have learned how to express numbers, operations, and variables. What about words? In Python, anything that goes between 'single' or "double" quotes is considered a string. Strings are commonly used to express words, but they have many other uses, including displaying information to the user and retrieving information from a user.
Examples include 'hello', "hello", 'HELLoo00', '12345', and 'fun_characters: !@ #$%^&*('.
In this section, you will gain proficiency with strings by examining string methods, string concatenation, and useful built-in functions including print()
and len()
with a wide range of examples.
String Syntax
Although strings may use single or double quotes, a given string must be internally consistent. That is, if a string starts with a single quote, it must end with a single quote. The same is true of double quotes.
You can take a look at valid and invalid strings in Exercise 7, String Error Syntax.
Exercise 7: String Error Syntax
The goal of this exercise is to learn appropriate string syntax:
- Open a Jupyter Notebook.
- Enter a valid string:
bookstore = 'City Lights'
- Now enter an invalid string:
bookstore = 'City Lights"
You should get the following output:
Figure 1.7: Output with invalid string format
If you start with a single quote, you must end with a single quote. Since the string has not been completed, you receive a syntax error.
- Now you need to enter a valid string format again, as in the following code snippet:
bookstore = "Moe's"
This is okay. The string starts and ends with double quotes. Anything can be inside the quotation marks, except for more quotation marks.
- Now add the invalid string again:
bookstore = 'Moe's'
You should get the following output:

Figure 1.8: Output with the invalid string
This is a problem. You started and ended with single quotes, and then you added an s
and another single quote.
A couple of questions arise. The first is whether single or double quotes should be used. The answer is that it depends on developer preference. Double quotes are more traditional, and they can be used to avoid potentially problematic situations such as the aforementioned Moe's
example. Single quotes eliminate the need to press the Shift
key.
In this exercise, you have learned the correct and incorrect ways of assigning strings to variables, including single and double-quotes.
Python uses the backslash character, \
, called an escape sequence in strings, to allow for the insertion of any type of quote inside of strings. The character that follows the backslash in an escape sequence may be interpreted as mentioned in Python's official documentation, which follows. Of particular note is \n
, which is used to create a new line:
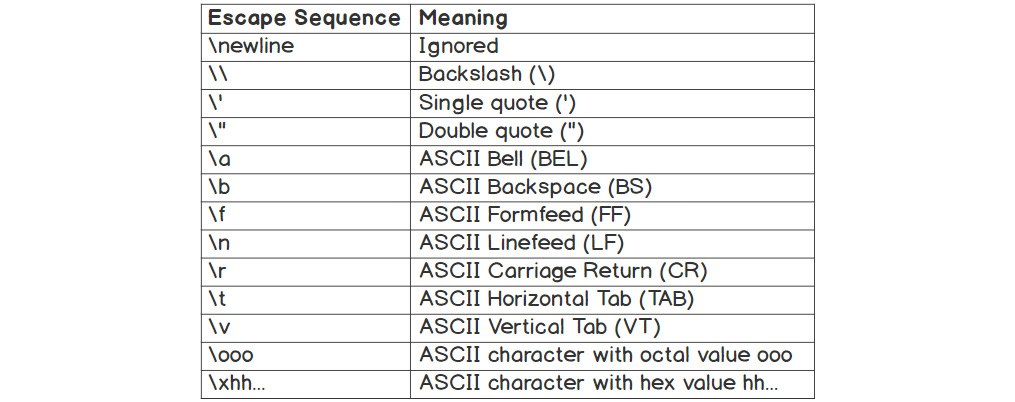
Figure 1.9: Escape sequences and their meaning
Note
For more information on strings, you can refer to https://docs.python.org/2.0/ref/strings.html.
Escape Sequences with Quotes
Here is how an escape sequence works with quotes. The backslash overrides the single quote as an end quote and allows it to be interpreted as a string character:
bookstore = 'Moe\'s'
Multi-Line Strings
Short strings always display nicely but, what about multi-line strings? It can be cumbersome to define a paragraph variable that includes a string over multiple lines. In some IDEs, the string may run off the screen, and it may be difficult to read. In addition, it might be advantageous to have line breaks at specific points for the user.
Note
Line breaks will not work inside single or double quotes.
When strings need to span multiple lines, Python provides triple quotes, using single or double quotation marks, as a nice option.
Here is an example of triple quotes ('''
) used to write a multi-line string:
vacation_note = ''' During our vacation to San Francisco, we waited in a long line by Powell St. Station to take the cable car. Tap dancers performed on wooden boards. By the time our cable car arrived, we started looking online for a good place to eat. We're heading to North Beach. '''
Note
Multi-line strings take on the same syntax as a docstring. The difference is that a docstring appears at the beginning of a document, and a multi-line string is defined within the program.
The print() Function
The print()
function is used to display information to the user, or to the developer. It's one of Python's most widely used built-in functions.
Exercise 8: Displaying Strings
In this exercise, you will learn different ways to display strings:
- Open a new Jupyter Notebook.
- Define a greeting variable with the value 'Hello'. Display the greeting using the
print()
function:greeting = 'Hello' print(greeting)
You should get the following output:
Hello
Hello
, as shown in the display, does not include single quotes. This is because theprint()
function is generally intended for the user to print the output.Note
The quotes are for developer syntax, not user syntax.
- Display the value of
greeting
without using theprint()
function:greeting
You should get the following output:
'Hello'
When we input
greeting
without theprint()
function, we are obtaining the encoded value, hence the quotes. - Consider the following sequence of code in a single cell in a Jupyter Notebook:
spanish_greeting = 'Hola.' spanish_greeting arabic_greeting = 'Ahlan wa sahlan.'
When the preceding cell is run, the preceding code does not display
spanish_greeting
. If the code were run on a terminal as three separate lines, it would displayHola.
, the string assigned tospanish_greeting
. The same would be true if the preceding sequence of code were run in three separate cells in a Jupyter Notebook. For consistency, it's useful to useprint()
any time information should be displayed. - Display the Spanish greeting:
spanish_greeting = 'Hola.' print(spanish_greeting)
You should get the following output:
Hola.
- Now, display the Arabic greeting message, as mentioned in the following code snippet:
arabic_greeting = 'Ahlan wa sahlan.' print(arabic_greeting)
You should get the following output:
Ahlan wa sahlan.
The compiler runs through each line in order. Every time it arrives at
print()
, it displays information.
In this exercise, you have learned different ways to display strings, including the print()
function. You will use the print()
function very frequently as a developer.
String Operations and Concatenation
The multiplication and addition operators work with strings as well. In particular, the +
operator combines two strings into one and is referred to as string concatenation. The *
operator, for multiplication, repeats a string. In the following exercise, you will be looking at string concatenation in our string samples.
Exercise 9: String Concatenation
In this exercise, you will learn how to combine strings using string concatenation:
- Open a new Jupyter Notebook.
- Combine the
spanish_greeting
we used in Exercise 8, Displaying Strings, with 'Senor.' using the+
operator and display the results:spanish_greeting = 'Hola' print(spanish_greeting + 'Senor.')
You should get the following output:
HolaSenor.
Notice that there are no spaces between
greeting
and name. If we want spaces between strings, we need to explicitly add them. - Now, combine
spanish_greeting
with 'Senor.' using the+
operator, but this time, include a space:spanish_greeting = 'Hola ' print(spanish_greeting + 'Senor.')
You should get the following output:
Hola Senor.
- Display the greeting 5 times using the
*
multiplication operator:greeting = 'Hello' print(greeting * 5)
You should get the following output:
HelloHelloHelloHelloHello
By completing this exercise successfully, you have combined strings using string concatenation using the +
and *
operators.